用C语言实现:定义商品信息结构体,包括商品名称、价格、数量、图片路径等属性,然后 使用链表来存储商品信息。怎么写
时间: 2024-02-07 19:02:28 浏览: 169
可以按照以下步骤来实现:
1. 定义商品信息结构体,包括商品名称(name)、价格(price)、数量(num)、图片路径(image_path)等属性:
```c
struct commodity {
char name[50];
float price;
int num;
char image_path[100];
};
```
2. 定义链表节点结构体,包括指向下一个节点的指针(next)和商品信息结构体(commodity):
```c
struct node {
struct commodity commodity;
struct node *next;
};
```
3. 定义链表的头指针(head),初始化为空指针(NULL):
```c
struct node *head = NULL;
```
4. 实现添加商品信息的函数(add_commodity),将商品信息插入链表的末尾:
```c
void add_commodity(struct commodity com) {
struct node *new_node = (struct node*)malloc(sizeof(struct node));
new_node->commodity = com;
new_node->next = NULL;
if(head == NULL) {
head = new_node;
return;
}
struct node *p = head;
while(p->next != NULL) {
p = p->next;
}
p->next = new_node;
}
```
5. 实现打印链表中所有商品信息的函数(print_commodity),遍历链表并打印每个节点的商品信息:
```c
void print_commodity() {
struct node *p = head;
while(p != NULL) {
printf("商品名称:%s\n", p->commodity.name);
printf("商品价格:%f\n", p->commodity.price);
printf("商品数量:%d\n", p->commodity.num);
printf("商品图片路径:%s\n", p->commodity.image_path);
printf("\n");
p = p->next;
}
}
```
6. 在主函数中,调用添加商品信息函数(add_commodity)和打印商品信息函数(print_commodity),可以按照以下方式添加和打印商品信息:
```c
int main() {
struct commodity com1 = {"商品1", 10.0, 100, "path1"};
struct commodity com2 = {"商品2", 20.0, 200, "path2"};
struct commodity com3 = {"商品3", 30.0, 300, "path3"};
add_commodity(com1);
add_commodity(com2);
add_commodity(com3);
print_commodity();
return 0;
}
```
阅读全文
相关推荐
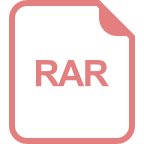
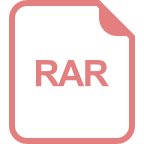
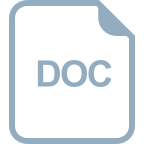
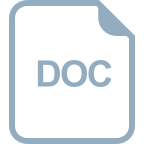
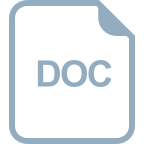
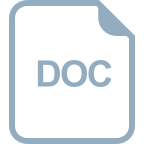
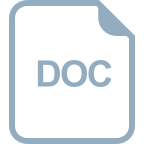
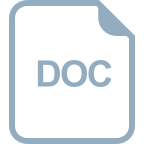
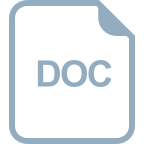
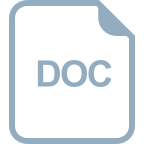
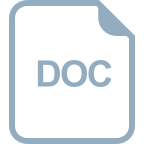

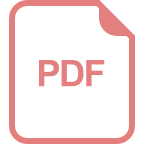
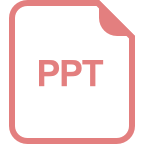
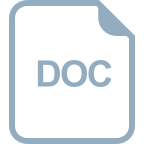
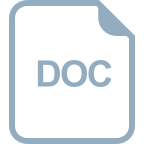
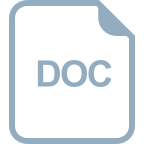
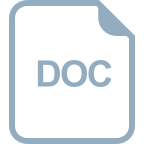