用C语言一个结构体链表并存储学号,姓名,成绩等数据
时间: 2023-03-15 20:21:33 浏览: 110
可以使用以下代码:
struct student
{
int num;
char name[20];
int grade;
struct student *next;
};struct student *head; // 定义链表头指针
head = (struct student *)malloc(sizeof(struct student)); // 为头指针申请内存空间
head->num = 0; // 初始化学号
head->grade = 0; // 初始化成绩
strcpy(head->name,""); // 初始化姓名
head->next = NULL; // 初始化指向下一节点的指针
相关问题
用C语言写一个结构体链表来存储学生的学号,姓名,成绩
可以使用以下代码:
struct student
{
int id;
char name[20];
float score;
struct student *next;
}; struct student *head = NULL; struct student *creat(int id, char name[], float score)
{
struct student *stu = (struct student*)malloc(sizeof(struct student));
if (stu == NULL)
{
return NULL;
}
stu->id = id;
strcpy(stu->name, name);
stu->score = score;
stu->next = NULL;
return stu;
} void insert(struct student *head, struct student *stu)
{
struct student *p = head;
while (p->next != NULL)
{
p = p->next;
}
p->next = stu;
}
构造结构体链表,结构体内容包括学号姓名和成绩c语言源码
要构造结构体链表,首先需要定义结构体的内容,包括学号、姓名和成绩。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体
typedef struct student {
int id;
char name[20];
int score;
struct student* next;
} Student;
int main() {
int n;
printf("请输入学生人数: ");
scanf("%d", &n);
Student* head = NULL;
Student* tail = NULL;
// 输入学生信息并构造链表
for (int i = 0; i < n; i++) {
printf("\n请输入第%d个学生的学号: ", i+1);
int id;
scanf("%d", &id);
printf("请输入姓名: ");
char name[20];
scanf("%s", name);
printf("请输入成绩: ");
int score;
scanf("%d", &score);
// 创建新的学生结点
Student* newStudent = (Student*)malloc(sizeof(Student));
newStudent->id = id;
strcpy(newStudent->name, name);
newStudent->score = score;
newStudent->next = NULL;
// 将新的学生结点加入链表
if (head == NULL) {
head = newStudent;
tail = newStudent;
} else {
tail->next = newStudent;
tail = tail->next;
}
}
// 遍历链表并打印学生信息
printf("\n学生信息如下:\n");
Student* current = head;
while (current != NULL) {
printf("学号: %d\n", current->id);
printf("姓名: %s\n", current->name);
printf("成绩: %d\n", current->score);
printf("\n");
current = current->next;
}
// 释放链表内存
while (head != NULL) {
Student* temp = head;
head = head->next;
free(temp);
}
return 0;
}
```
以上是一个通过输入学生信息构造结构体链表的程序。首先输入学生人数,然后按照提示输入每个学生的学号、姓名和成绩。程序将会创建新的学生结点,并通过将结点链接在一起构造链表。最后,程序将会遍历链表并打印每个学生的学号、姓名和成绩。最后释放链表内存,结束程序的执行。
相关推荐
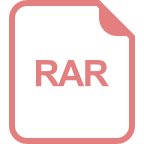
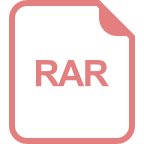












