请用c语言编写程序实现链表的建立、插入、删除、遍历的基本运算,其中顺序表中每一个数据元素的类型定义为学生信息的结构体,包括学号、姓名和成绩。
时间: 2023-05-19 22:01:27 浏览: 52
好的,以下是实现链表基本运算的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生信息结构体
typedef struct student {
int id;
char name[20];
float score;
struct student *next;
} Student;
// 创建链表
Student *createList() {
Student *head = (Student *)malloc(sizeof(Student));
head->next = NULL;
return head;
}
// 插入节点
void insertNode(Student *head, int id, char *name, float score) {
Student *p = head;
while (p->next != NULL) {
p = p->next;
}
Student *newNode = (Student *)malloc(sizeof(Student));
newNode->id = id;
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = NULL;
p->next = newNode;
}
// 删除节点
void deleteNode(Student *head, int id) {
Student *p = head;
while (p->next != NULL && p->next->id != id) {
p = p->next;
}
if (p->next == NULL) {
printf("未找到该学生信息!\n");
return;
}
Student *temp = p->next;
p->next = temp->next;
free(temp);
}
// 遍历链表
void traverseList(Student *head) {
Student *p = head->next;
while (p != NULL) {
printf("学号:%d\t姓名:%s\t成绩:%.2f\n", p->id, p->name, p->score);
p = p->next;
}
}
int main() {
Student *head = createList();
insertNode(head, 1001, "张三", 90.5);
insertNode(head, 1002, "李四", 85.0);
insertNode(head, 1003, "王五", 92.5);
printf("插入节点后的链表:\n");
traverseList(head);
deleteNode(head, 1002);
printf("删除节点后的链表:\n");
traverseList(head);
return 0;
}
```
希望这个代码能够满足您的需求!
相关推荐
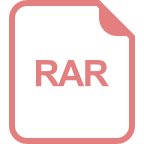
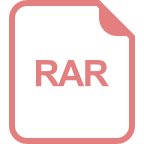








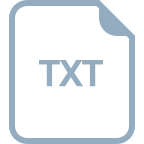
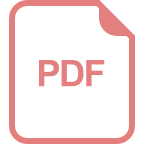
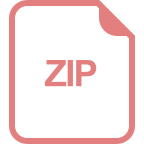
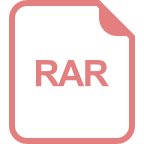
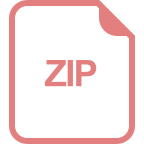
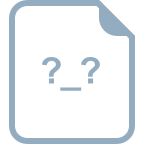
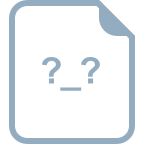
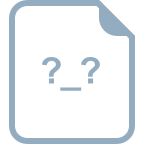