if(RTC->ISR & RTC_ISR_ALRAF) { RTC->ISR &= ~RTC_ISR_ALRAF; // Check if data was received during sleep and handle accordingly if(dataReceived) { // Handle data transfer dataReceived = 0;//重置标志位 } else { // Delay for DATA_TIMEOUT seconds and then reset the device for(int i = 0; i < DATA_TIMEOUT; i++) { HAL_Delay(1000); } NVIC_SystemReset();//系统重启 } }
时间: 2024-02-10 16:21:18 浏览: 211
这段代码的作用是检测RTC的ALARM A标志位是否被置位,如果被置位则表示已经到达了ALARM A设定的时间。当ALARM A触发时,会根据是否有数据被接收到来进行相应的处理。
具体来说,这段代码的执行流程如下:
1. 检测RTC的ALARM A标志位是否被置位。
2. 如果被置位,则清除ALARM A标志位。
3. 检测是否有数据被接收到。
4. 如果有数据被接收到,则进行数据传输的处理。
5. 如果没有数据被接收到,则延时DATA_TIMEOUT秒后重启系统。
需要注意的是,延时的时间为DATA_TIMEOUT秒,可以根据实际需求进行修改。同时,系统重启会导致所有的数据和状态都被清除,因此需要谨慎使用。
相关问题
如何把 if(RTC->ISR & RTC_ISR_ALRAF) { RTC->ISR &= ~RTC_ISR_ALRAF; // Check if data was received during sleep and handle accordingly if(dataReceived) { // Handle data transfer dataReceived = 0;//重置标志位 } else { // Delay for DATA_TIMEOUT seconds and then reset the device for(int i = 0; i < DATA_TIMEOUT; i++) { HAL_Delay(1000); } NVIC_SystemReset();//系统重启 } }和if(LPUART1->ISR & USART_ISR_RXNE) // 检测 LPUART1 接收数据的标志位是否被置位 { char receivedData = LPUART1->RDR; // 取出接收到的字符 if(receivedData == targetChar) // 判断取出的字符是否与目标字符一致 { printf("Received target character: %c\n", targetChar); // 输出一条信息 } }两段代码结合起来
这两段代码可以结合起来实现一个功能:在接收到特定字符时,重置设备。具体实现方法如下:
1. 在程序中定义一个标志位 `char targetChar = 'R';`,表示接收到该字符时需要重置设备。
2. 在主函数中加入以下代码:
```
int main()
{
// 其他初始化代码
while(1)
{
if(RTC->ISR & RTC_ISR_ALRAF)
{
RTC->ISR &= ~RTC_ISR_ALRAF;
// Check if data was received during sleep and handle accordingly
if(dataReceived)
{
// Handle data transfer
dataReceived = 0;
}
else
{
// Delay for DATA_TIMEOUT seconds and then reset the device
for(int i = 0; i < DATA_TIMEOUT; i++)
{
HAL_Delay(1000);
}
NVIC_SystemReset();
}
}
if(LPUART1->ISR & USART_ISR_RXNE)
{
char receivedData = LPUART1->RDR;
if(receivedData == targetChar)
{
printf("Received target character: %c\n", targetChar);
NVIC_SystemReset();
}
}
// Other code
}
}
```
这段代码会不断循环执行,检测是否收到特定字符或者 RTC 的 ALRAF 标志位是否被置位。如果收到特定字符,则输出一条信息并重置设备;如果 RTC 的 ALRAF 标志位被置位,则在延时一定时间后重置设备。
static __IO uint32_t delay_ms_ticks; static inline void delay_init(void) { SysTick_Config(SystemCoreClock/1000); } static inline void delay_ms(uint16_t ms) { delay_ms_ticks = ms; while(delay_ms_ticks); } __weak void SysTick_Handler() { if (delay_ms_ticks > 0) { delay_ms_ticks--; } }改为使用外部32.768KHz晶振的延时函数
首先,需要明确的是使用外部32.768KHz晶振的延时函数需要使用RTC定时器,因为RTC定时器使用的是低速外部晶振。以下是修改后的代码:
```c
static __IO uint32_t delay_ms_ticks;
static inline void delay_init(void) {
RCC->APB1ENR1 |= RCC_APB1ENR1_PWREN; // Enable power interface clock
PWR->CR1 |= PWR_CR1_DBP; // Enable access to RTC, backup and power registers
RCC->BDCR |= RCC_BDCR_RTCEN; // Enable RTC clock
RCC->BDCR |= RCC_BDCR_RTCSEL_LSE; // Select low speed external oscillator as RTC clock
RTC->WPR = 0xCA; // Unlock write protection
RTC->WPR = 0x53;
RTC->ISR |= RTC_ISR_INIT; // Enter initialization mode
while (!(RTC->ISR & RTC_ISR_INITF)); // Wait for initialization flag to be set
RTC->PRER = (127 << 16) | (255); // Set prescaler values for 32.768KHz oscillator
RTC->ISR &= ~RTC_ISR_INIT; // Exit initialization mode
RTC->WPR = 0xFF; // Lock write protection
}
static inline void delay_ms(uint16_t ms) {
delay_ms_ticks = ms;
while (delay_ms_ticks);
}
__weak void RTC_WKUP_IRQHandler() {
if (delay_ms_ticks > 0) {
delay_ms_ticks--;
}
}
```
在上述代码中,我们首先使能了PWR、RTC和BKP的时钟,并且解锁了RTC写保护。然后选择了低速外部晶振作为RTC时钟源,并且设置了对应的预分频器值。最后,我们将写保护锁定,并在RTC的WKUP中断句柄中进行延时操作。需要注意的是,在这里我们使用了RTC的WKUP中断而不是SysTick中断来进行延时,因为我们现在使用的是RTC定时器。
阅读全文
相关推荐
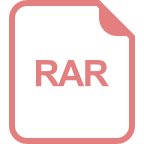
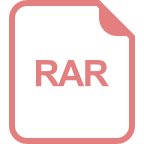
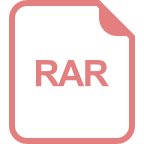
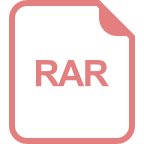
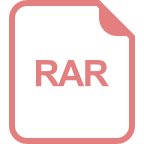
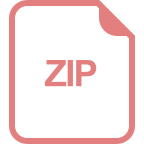
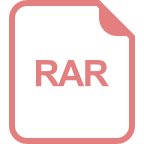
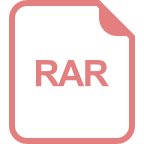
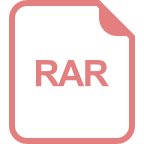
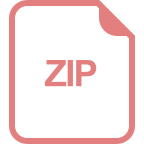
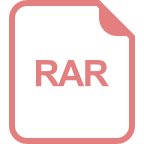
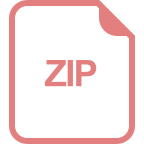
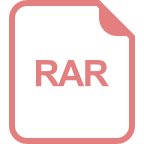


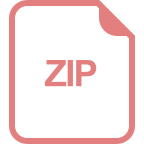