stm32单片机简单加法计算器系统代码
时间: 2024-06-13 14:01:33 浏览: 11
好的,STM32是一款广泛使用的单片机,其代码编写采用C语言或汇编语言。以下是一个简单的加法计算器的代码示例,适用于STM32F1系列单片机:
```
#include "stm32f10x.h"
int main(void)
{
// 初始化GPIO和USART
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 配置USART1 Tx (PA9)引脚为复用功能推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1 Rx (PA10)引脚为浮空输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1初始化配置
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 开启USART1
USART_Cmd(USART1, ENABLE);
// 定义两个变量,用于存放两个数值
uint8_t num1, num2;
while (1)
{
// 接收第一个数值
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
num1 = USART_ReceiveData(USART1);
// 接收第二个数值
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
num2 = USART_ReceiveData(USART1);
// 计算结果并通过USART发送回去
uint8_t result = num1 + num2;
USART_SendData(USART1, result);
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
}
```
相关问题:
1. STM32单片机适合做哪些项目?
2. 如何调试STM32单片机程序?
3. 如何在STM32单片机上使用PWM输出?
相关推荐
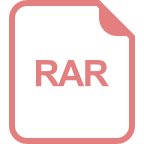
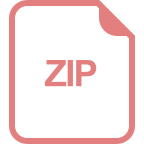
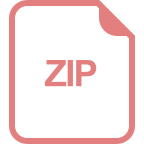
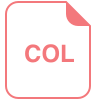
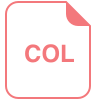
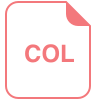
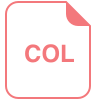
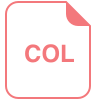









