如何从python内置函数read()返回值中提取像素数据
时间: 2024-03-21 07:43:19 浏览: 16
使用Python内置函数read()函数读取图像文件时,返回的是二进制数据,需要对其进行解析才能获取像素数据。对于不同的图像格式,解析方式也不同。
以BMP格式为例,BMP格式的文件头部分包含了一些文件信息和图像属性信息,需要先解析出这些信息,才能获取像素数据。
下面是一个示例代码,用于从BMP格式的文件中读取像素数据:
```python
with open('test.bmp', 'rb') as f:
# 解析BMP文件头信息
header = f.read(54) # BMP文件头部分54个字节
width = header[18:22] # 图像宽度信息
height = header[22:26] # 图像高度信息
bpp = header[28:30] # 每像素位数信息
data_offset = header[10:14] # 数据偏移量信息
# 计算像素数据的大小
data_size = int.from_bytes(header[34:38], byteorder='little')
# 读取像素数据
f.seek(int.from_bytes(data_offset, byteorder='little'))
data = f.read(data_size)
# 将像素数据转换为二维数组
pixels = []
for i in range(int.from_bytes(height, byteorder='little')):
row = []
for j in range(int.from_bytes(width, byteorder='little')):
if int.from_bytes(bpp, byteorder='little') == 24:
# 每像素24位
b = data[i * int.from_bytes(width, byteorder='little') * 3 + j * 3]
g = data[i * int.from_bytes(width, byteorder='little') * 3 + j * 3 + 1]
r = data[i * int.from_bytes(width, byteorder='little') * 3 + j * 3 + 2]
row.append((r, g, b))
elif int.from_bytes(bpp, byteorder='little') == 32:
# 每像素32位
b = data[i * int.from_bytes(width, byteorder='little') * 4 + j * 4]
g = data[i * int.from_bytes(width, byteorder='little') * 4 + j * 4 + 1]
r = data[i * int.from_bytes(width, byteorder='little') * 4 + j * 4 + 2]
row.append((r, g, b))
pixels.append(row)
# 打印像素数据
print(pixels)
```
在上面的代码中,我们首先使用Python内置函数open()函数以二进制模式打开BMP格式的文件,然后读取文件头部分的信息,包括图像的宽度、高度、每像素位数等信息。接着,根据数据偏移量信息,计算出像素数据的大小,并读取像素数据。最后,我们将像素数据转换为二维数组,并打印出来。
需要注意的是,不同图像格式的解析方式不同,上面的代码仅适用于BMP格式的图像文件。对于其他格式的图像文件,需要使用相应的解析方法。
相关推荐
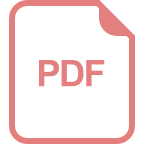
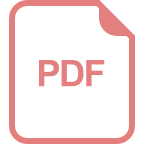
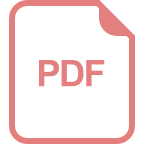
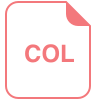
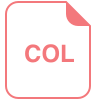












