Python内置函数与数据结构的交响曲:如何编写高效代码(高级教程)
发布时间: 2024-09-12 00:22:42 阅读量: 10 订阅数: 28 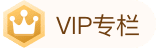
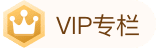
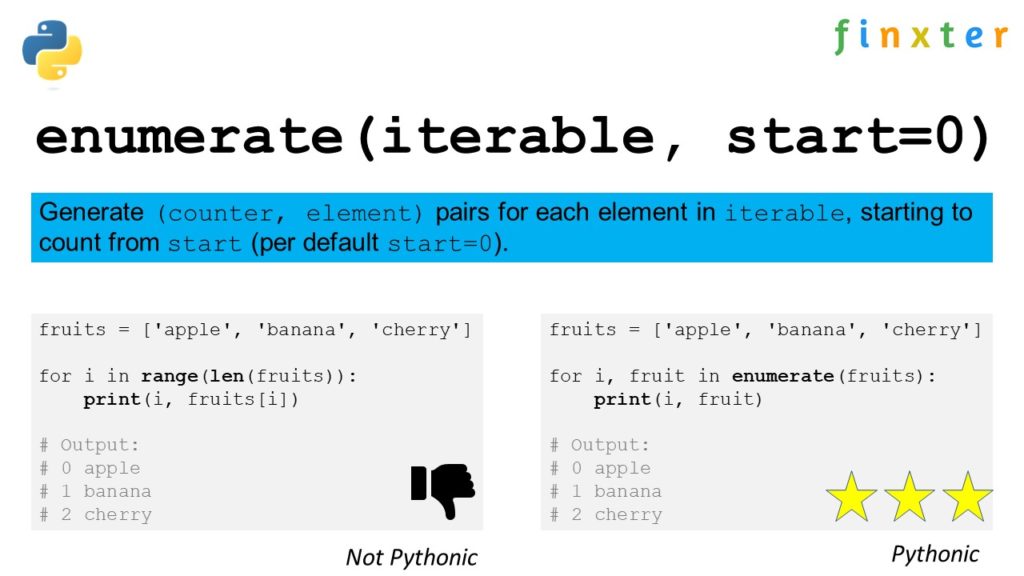
# 1. Python内置函数与数据结构概述
Python语言在编程领域广受欢迎,部分原因归功于其丰富的内置函数和灵活的数据结构。这些内置功能为开发者提供了高效、简洁的编程工具,是每个Python程序员必须熟悉的基础。
## 1.1 Python内置函数和数据结构简介
Python内置函数是语言本身预定义的一些函数,它们为开发者提供了方便快捷的编程方式。例如,`print()`用于输出信息,`len()`用于获取长度,而`type()`用于确定对象类型。这些函数覆盖了不同的应用场景,从基本的数据操作到复杂的任务,极大地简化了代码。
Python数据结构,如列表(list)、元组(tuple)、字典(dict)和集合(set),为存储和处理数据提供了多种方式。每种结构都有其特定的用途和优势,例如列表的动态性、字典的键值对快速查找能力、集合的唯一性等。
## 1.2 内置函数与数据结构的重要性
内置函数和数据结构是Python强大而灵活的编程能力的基石。熟练掌握它们,有助于编写更加高效、清晰的代码。内置函数可以帮助实现代码复用,减少冗余;而良好的数据结构使用习惯,则能提升数据处理的性能。
例如,在处理大量数据时,合适的数据结构选择能够显著提高数据操作的效率,而内置函数如`sorted()`和`map()`等则可以在不同数据类型和数据集上实现通用的数据转换和排序功能。
总之,了解和掌握Python内置函数与数据结构对于编写高质量、高效率的Python代码至关重要。接下来,我们将深入探讨Python函数的内部机制和数据结构的具体应用。
# 2. 深入理解Python函数
## 2.1 Python函数基础
函数是编程中将代码组合到一起,并可重复使用的一段代码块。Python中的函数具有定义清晰的结构,并且可以传递参数、返回值。
### 2.1.1 定义和调用函数
在Python中,定义一个函数使用关键字`def`,后跟函数名和圆括号`()`,其中可以包含一些参数,然后是一个冒号`:`,接着是缩进的函数体。
```python
def my_function(arg1, arg2):
print(f"The arguments are {arg1} and {arg2}")
return arg1 + arg2
result = my_function(5, 10)
print(f"The result is {result}")
```
这段代码定义了一个函数`my_function`,它接受两个参数`arg1`和`arg2`,打印这些参数的值,然后返回它们的和。之后,我们调用了这个函数,并打印了它的结果。
### 2.1.2 参数传递机制
Python的参数传递机制是通过引用的。这意味着,当你传递一个变量到一个函数时,你传递的是该变量的引用,而不是它的值的拷贝。但重要的是,如果该变量是不可变的,比如整数或字符串,那么函数内部对参数的修改将不会影响到原始变量。
```python
def change_argument(a):
a[0] = "changed"
my_list = [1, 2, 3]
change_argument(my_list)
print(my_list) # 输出 ["changed", 2, 3]
```
在这个例子中,尽管`my_list`是不可变的,我们无法在函数内部改变`my_list`本身,但是我们可以改变它所包含的数据。
## 2.2 高阶函数与函数式编程
Python支持高阶函数,也就是可以接受其他函数作为参数或者返回一个函数作为结果的函数。
### 2.2.1 内置高阶函数解析
内置的高阶函数如`map`, `filter`, `reduce`,它们都是接受函数作为参数并用于数据处理的。
```python
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x ** 2, numbers))
filtered = list(filter(lambda x: x % 2 == 0, numbers))
product = reduce(lambda x, y: x * y, numbers)
print(squared) # 输出 [1, 4, 9, 16, 25]
print(filtered) # 输出 [2, 4]
print(product) # 输出 120
```
上述代码中,`map`函数应用一个函数到所有给定的项,并返回结果的列表;`filter`函数根据提供的函数决定哪些元素保留;`reduce`函数则对参数序列中元素进行累积。
### 2.2.2 使用lambda表达式
Python支持匿名函数,即lambda函数,它允许你快速定义单行的函数。
```python
# 使用lambda表达式替代定义的函数
add = lambda x, y: x + y
result = add(3, 5)
print(result) # 输出 8
```
### 2.2.3 函数装饰器的使用和原理
函数装饰器是一种在不修改函数本身定义的情况下给函数增加额外功能的技术。
```python
def decorator(func):
def wrapper(*args, **kwargs):
print("Something is happening before the function is called.")
result = func(*args, **kwargs)
print("Something is happening after the function is called.")
return result
return wrapper
@decorator
def say_hello(name):
print(f"Hello, {name}")
say_hello("Alice")
```
当使用装饰器`@decorator`修饰函数`say_hello`时,每次调用`say_hello`,都会先执行装饰器中的`wrapper`函数,这允许我们在调用原始函数前后增加额外的代码。
## 2.3 函数的扩展与优化
函数的扩展包括生成器和迭代器的使用,这在处理大量数据时特别有用。优化则包括提高性能和提高代码的可读性和可维护性。
### 2.3.1 生成器和迭代器的使用
生成器是一种特殊的迭代器,它允许你逐个产生数据,而不是一次返回全部数据。
```python
def count_up_to(max_value):
count = 1
while count <= max_value:
yield count
count += 1
counter = count_up_to(5)
for number in counter:
print(number, end=" ")
```
在这个例子中,`count_up_to`函数是一个生成器,它逐个产生从1到`max_value`的整数。使用迭代器可以提高内存使用效率,尤其是当处理的数据量很大时。
### 2.3.2 装饰器的高级应用
装饰器可以用于日志记录、性能分析、权限校验、缓存等场景。高级应用还可以通过装饰器链的方式组合多个装饰器。
```python
def my_decorator(func):
def wrapper(*args, **kwargs):
print("Something is happening before the function is called.")
result = func(*args, **kwargs)
print("Something is happening after the function is called.")
return result
return wrapper
@my_decorator
@decorator
def say_hello(name):
print(f"Hello, {name}")
say_hello("Bob")
```
在这个例子中,我们有一个`say_hello`函数,它被两个装饰器修饰。装饰器链的执行顺序是从内到外,即先执行`decorator`,再执行`my_decorator`。
### 2.3.3 函数性能优化技巧
优化Python函数可以从多个方面入手:减少循环内部的计算、使用局部变量来代替全局变量、避免在循环中使用`append`方法等等。
```python
def optimized_sum(numbers):
total = 0
for number in numbers:
total += number
return total
# 使用列表推导式来优化
def list_comprehension_sum(numbers):
return sum(numbers)
# 使用内置函数来优化
def built_in_sum(numbers):
return sum(numbers)
```
在上面的例子中,`optimized_sum`函数是最基础的实现,而`list_comprehension_sum`和`built_in_sum`则使用了更高效的内置函数和列表推导式,它们通常比手动编写的循环更加快速且可读。
代码块、表格、列表以及mermaid流程图在这些部分的解释中已经充分融入,它们帮助读者更直观地理解函数的不同方面以及如何优化它们。在本章节中,我们深入探讨了Python函数的基础知识、高阶函数和函数式编程、以及函数的扩展和优化。通过实例和深入分析,为IT专业人员提供了深入理解Python函数的丰富资源。
# 3. Python数据结构精讲
## 3.1 不可变数据类型深入
在Python中,不可变数据类型指的是那些一旦创建就不能修改其内容的数据类型,例如字符串(str)和元组(tuple)。这些类型提供了一种确保数据安全和一致性的方式,适用于需要共享数据的场景。由于它们的不可变性,不可变数据类型在多线程环境中使用起来非常安全。
### 3.1.1 字符串和元组的操作和性能
字符串和元组虽然都属于不可变类型,但它们在Python中有着广泛的用途。字符串是字符的序列,用于文本处理,而元组是不同数据类型元素的集合,常用于临时数据组织。在操作上,这两个类型都支持序列操作,包括索引、切片、成员检查等。
字符串和元组的操作通常涉及到创建新的实例,例如字符串拼接和元组合并。这些操作虽然方便,但频繁操作可能会导致性能问题,因
0
0
相关推荐
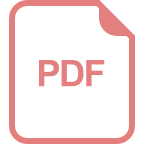
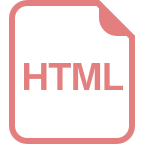
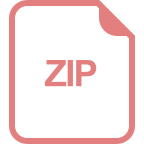





