Python内置函数在数据结构中的作用与重要性:为什么每个开发者都该掌握(必知必会)
发布时间: 2024-09-12 00:58:45 阅读量: 14 订阅数: 28 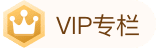
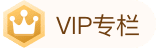
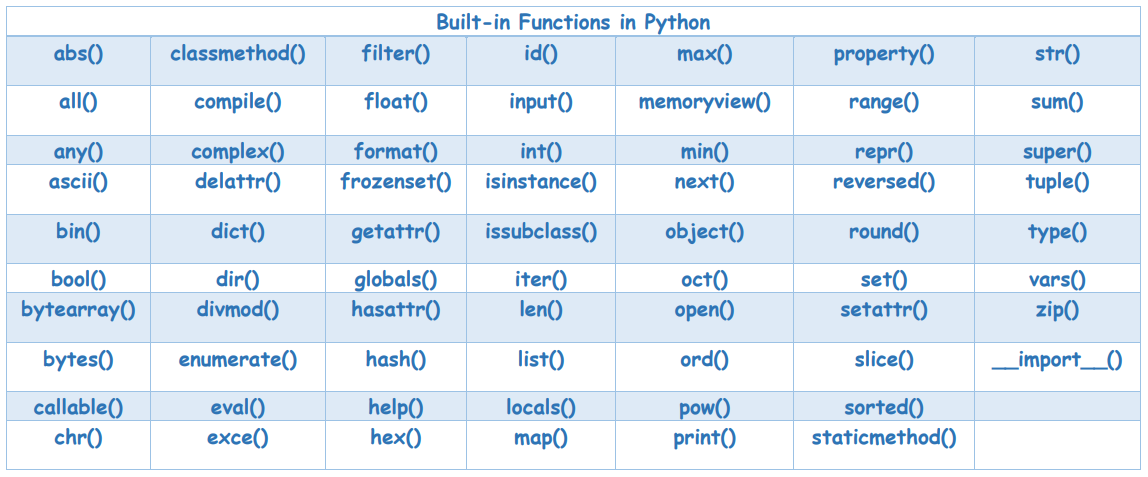
# 1. Python内置函数概述
Python作为一门功能丰富的编程语言,内置了大量的函数以方便开发者使用。内置函数主要指的是在Python标准库中已经定义好的函数,这些函数可以直接调用,无需额外安装或导入模块。内置函数的范围涵盖了从基础数据类型操作到复杂逻辑处理等多个层面,例如`print()`, `len()`, `range()`, `sorted()`等。这些函数的使用不仅提高了开发效率,而且还有助于代码的简洁性和可读性。
在深入探讨内置函数的具体应用之前,我们有必要先对Python的内置函数有一个大致的了解。根据不同的数据结构和操作需求,内置函数可以大致分为几类,如集合操作函数、字典处理函数、列表和元组操作函数等。掌握这些分类有助于在编程时快速找到合适的工具解决问题。
本章将对Python内置函数的基本概念进行介绍,概述它们的分类、特点以及使用场景。通过本章学习,读者将为后续章节中具体内置函数的深入分析和应用打下坚实的基础。
# 2. 集合操作中的内置函数应用
### 2.1 集合的基本概念和操作
#### 2.1.1 集合的创建和基本操作
集合(Set)是Python中一种无序且唯一元素的集合数据类型。创建集合最简单的方法是使用大括号 `{}` 或者 `set()` 函数。
```python
# 使用大括号创建集合
fruits = {'apple', 'banana', 'cherry'}
print(fruits) # 输出: {'banana', 'cherry', 'apple'}
# 使用set函数创建集合
numbers = set([1, 2, 3, 3, 4, 5])
print(numbers) # 输出: {1, 2, 3, 4, 5}
```
需要注意的是,由于集合中的元素是唯一的,所以重复的元素会被自动去除。此外,集合是无序的,不能使用索引访问元素。
#### 2.1.2 集合的逻辑运算和成员关系
集合支持基本的逻辑运算,如并集、交集、差集和对称差集。这使得集合成为处理不相交数据集的理想选择。
```python
A = {1, 2, 3, 4}
B = {3, 4, 5, 6}
# 并集(Union): 所有在A或B中的元素
print(A | B) # 输出: {1, 2, 3, 4, 5, 6}
# 交集(Intersection): 同时在A和B中的元素
print(A & B) # 输出: {3, 4}
# 差集(Difference): 在A中但不在B中的元素
print(A - B) # 输出: {1, 2}
# 对称差集(Symmetric Difference): 在A或B中但不同时在A和B中的元素
print(A ^ B) # 输出: {1, 2, 5, 6}
```
集合还提供了成员关系操作符 `in` 和 `not in` 来检查元素是否存在于集合中。
### 2.2 内置函数在集合操作中的作用
#### 2.2.1 排序和计数函数
虽然集合是无序的,但我们可以使用 `sorted()` 函数对集合中的元素进行排序。此外,`len()` 函数可以用来计数集合中的元素数量。
```python
# 使用sorted()对集合进行排序
sorted_fruits = sorted(fruits)
print(sorted_fruits) # 输出: ['apple', 'banana', 'cherry']
# 使用len()函数计算集合大小
print(len(fruits)) # 输出集合中元素的个数
```
#### 2.2.2 映射和过滤函数
在Python中,虽然集合不支持像列表那样的索引操作,但我们可以使用集合推导式(set comprehension)来过滤和映射集合中的元素。
```python
# 使用集合推导式过滤集合
even_numbers = {x for x in numbers if x % 2 == 0}
print(even_numbers) # 输出: {2, 4}
# 使用集合推导式映射集合
squared_numbers = {x * x for x in numbers}
print(squared_numbers) # 输出: {1, 4, 9, 16, 25}
```
#### 2.2.3 集合转换函数
集合可以转换为列表和元组,反之亦然。这为集合提供了与其他数据结构交互的能力。
```python
# 将集合转换为列表
fruits_list = list(fruits)
print(fruits_list) # 输出: ['apple', 'banana', 'cherry']
# 将列表转换回集合
fruits_set = set(fruits_list)
print(fruits_set) # 输出: {'apple', 'banana', 'cherry'}
# 将集合转换为元组
fruits_tuple = tuple(fruits)
print(fruits_tuple) # 输出: ('apple', 'banana', 'cherry')
# 将元组转换为集合
fruits_set = set(fruits_tuple)
print(fruits_set) # 输出: {'apple', 'banana', 'cherry'}
```
通过对集合操作中的内置函数进行应用,我们可以更好地管理数据集,执行集合运算,并解决各种编程问题。这些内置函数不仅简化了代码,还提高了效率和可读性。在下一节中,我们将深入了解字典处理中的内置函数应用。
# 3. 字典处理中的内置函数应用
#### 3.1 字典数据结构的特性
##### 3.1.1 字典的创建和访问
在Python中,字典是一种可变的键值对集合,使用大括号`{}`或者`dict()`函数来创建。字典的键必须是不可变类型,如字符串、数字或元组,而值可以是任意数据类型。
```python
# 通过大括号创建字典
person = {'name': 'Alice', 'age': 30, 'city': 'New York'}
# 访问字典中的值
print(person['name']) # 输出: Alice
```
字典的创建可以通过`dict()`函数,也可以通过关键字参数的方式:
```python
# 使用dict函数
another_person = dict(name='Bob', age=25)
# 使用关键字参数
another_person = dict(zip(['name', 'age', 'city'], ['Charlie', 35, 'Chicago']))
```
访问字典中的值需要注意的是,如果键不存在于字典中,将会抛出一个`KeyError`异常。为了避免这种情况,可以使用`get()`方法:
```python
# 使用get方法访问,如果键不存在则返回None
print(person.get('gender')) # 输出: None
# 也可以指定返回的默认值
print(another_person.get('gender', 'Unknown')) # 输出: Unknown
```
##### 3.1.2 字典的修改和合并
字典是可变的,因此可以修改其键值对。可以通过赋值的方式修改已有的键值对,或者添加新的键值对。
```python
# 修改字典中的键值对
person['age'] = 31
# 添加新的键值对
person['gender'] = 'Female'
```
合并两个字典可以通过多种方式实现。在Python 3.5及以上版本,可以直接使用`|`运算符:
```python
# Python 3.5及以上版本可以使用 | 运算符合并字典
combined_dict = person | another_person
```
对于旧版本的Python,需要使用`update()`方法或者`dict()`函数:
```python
# 使用update方法合并字典
person.update(another_person)
# 使用dict函数和**操作符
co
```
0
0
相关推荐
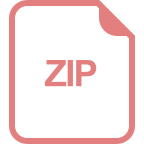
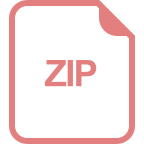
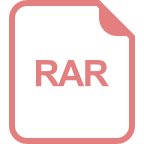





