用Visual Studio Code帮我写一个日历页面
时间: 2024-04-06 15:08:42 浏览: 22
好的,我可以为您提供一些指导。首先,您需要使用 HTML、CSS 和 JavaScript 来创建日历页面。
以下是一个基本的 HTML 结构:
```
<!DOCTYPE html>
<html>
<head>
<title>Calendar Page</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="calendar"></div>
<script src="script.js"></script>
</body>
</html>
```
这里我们引入了样式表和 JavaScript 脚本。接下来,我们需要在 `div` 标签内创建一个表格,用于显示日历。
在 `script.js` 文件中,您可以使用 JavaScript 来动态生成日历。以下是一个示例代码:
```
const date = new Date();
const year = date.getFullYear();
const month = date.getMonth();
const calendar = document.getElementById("calendar");
// Create table element
const table = document.createElement("table");
// Create table head element
const thead = document.createElement("thead");
const tr = document.createElement("tr");
const th1 = document.createElement("th");
const th2 = document.createElement("th");
const th3 = document.createElement("th");
const th4 = document.createElement("th");
const th5 = document.createElement("th");
const th6 = document.createElement("th");
const th7 = document.createElement("th");
th1.innerHTML = "Sun";
th2.innerHTML = "Mon";
th3.innerHTML = "Tue";
th4.innerHTML = "Wed";
th5.innerHTML = "Thu";
th6.innerHTML = "Fri";
th7.innerHTML = "Sat";
tr.appendChild(th1);
tr.appendChild(th2);
tr.appendChild(th3);
tr.appendChild(th4);
tr.appendChild(th5);
tr.appendChild(th6);
tr.appendChild(th7);
thead.appendChild(tr);
table.appendChild(thead);
// Create table body element
const tbody = document.createElement("tbody");
let dateNum = 1;
let isCurrentMonth = false;
for (let i = 0; i < 6; i++) {
const tr = document.createElement("tr");
for (let j = 0; j < 7; j++) {
const td = document.createElement("td");
if (i === 0 && j < date.getDay()) {
td.innerHTML = "";
} else if (dateNum > daysInMonth(month, year)) {
break;
} else {
td.innerHTML = dateNum;
dateNum++;
if (dateNum === date.getDate() + 1 && month === date.getMonth() && !isCurrentMonth) {
td.classList.add("today");
}
if (dateNum === 1 && !isCurrentMonth) {
isCurrentMonth = true;
}
}
tr.appendChild(td);
}
tbody.appendChild(tr);
}
table.appendChild(tbody);
calendar.appendChild(table);
function daysInMonth(month, year) {
return new Date(year, month + 1, 0).getDate();
}
```
此代码会在 `div` 元素中动态生成一个表格,显示当前月份的日历。您可以根据需要进行修改和定制。
最后,您需要使用 CSS 来美化日历页面。以下是一个简单的样式表:
```
table {
width: 100%;
border-collapse: collapse;
}
thead th {
background-color: #f2f2f2;
border: 1px solid #ddd;
padding: 8px;
text-align: center;
}
td {
border: 1px solid #ddd;
padding: 8px;
text-align: center;
}
.today {
background-color: #4CAF50;
color: white;
}
```
此样式表会使表格看起来更加美观。您可以根据需要进行修改和定制。
希望这些指导能够帮助您创建一个基本的日历页面。
相关推荐
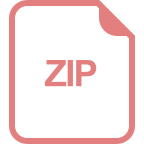
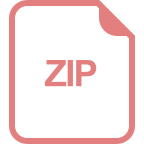
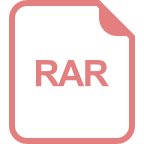















