openssl 编程对PKCS#12格式的证书进行验签
时间: 2024-02-23 12:59:35 浏览: 134
可以使用 OpenSSL 库中的 PKCS12_parse 函数来解析 PKCS#12 格式的证书,然后使用 X509_verify_cert 函数来验证证书链。以下是一个简单的示例代码:
```c
#include <openssl/pkcs12.h>
#include <openssl/x509_vfy.h>
int verify_pkcs12(const char *pkcs12_file, const char *password) {
PKCS12 *p12 = NULL;
EVP_PKEY *pkey = NULL;
X509 *cert = NULL;
STACK_OF(X509) *ca = NULL;
X509_STORE *store = NULL;
X509_STORE_CTX *ctx = NULL;
int ret = -1;
/* Load PKCS#12 file */
FILE *fp = fopen(pkcs12_file, "rb");
if (!fp) {
perror("fopen");
goto end;
}
p12 = d2i_PKCS12_fp(fp, NULL);
fclose(fp);
if (!p12) {
printf("Error loading PKCS#12 file\n");
ERR_print_errors_fp(stderr);
goto end;
}
/* Extract certificate and private key */
if (!PKCS12_parse(p12, password, &pkey, &cert, &ca)) {
printf("Error extracting certificate and private key\n");
ERR_print_errors_fp(stderr);
goto end;
}
/* Verify certificate chain */
store = X509_STORE_new();
if (!store) {
printf("Error creating X509 store\n");
ERR_print_errors_fp(stderr);
goto end;
}
for (int i = 0; i < sk_X509_num(ca); i++) {
X509_STORE_add_cert(store, sk_X509_value(ca, i));
}
X509_STORE_set_flags(store, X509_V_FLAG_CRL_CHECK | X509_V_FLAG_CRL_CHECK_ALL);
ctx = X509_STORE_CTX_new();
if (!ctx) {
printf("Error creating X509 store context\n");
ERR_print_errors_fp(stderr);
goto end;
}
if (X509_STORE_CTX_init(ctx, store, cert, ca) != 1) {
printf("Error initializing X509 store context\n");
ERR_print_errors_fp(stderr);
goto end;
}
if (X509_verify_cert(ctx) != 1) {
printf("Error verifying certificate chain\n");
ERR_print_errors_fp(stderr);
goto end;
}
/* Verification succeeded */
ret = 0;
end:
if (p12) PKCS12_free(p12);
if (pkey) EVP_PKEY_free(pkey);
if (cert) X509_free(cert);
if (ca) sk_X509_pop_free(ca, X509_free);
if (ctx) X509_STORE_CTX_free(ctx);
if (store) X509_STORE_free(store);
return ret;
}
```
该函数将返回 0 表示验证成功,-1 表示验证失败。
阅读全文
相关推荐
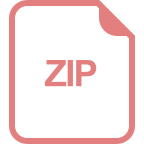
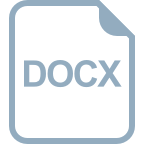
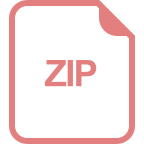
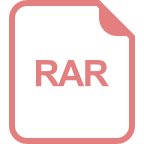
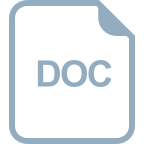
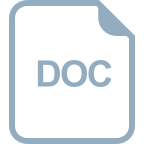
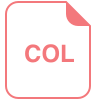

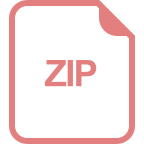
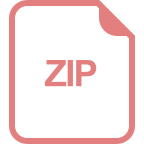
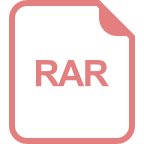
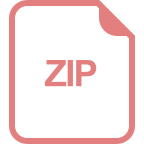
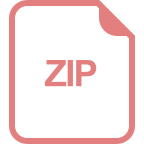
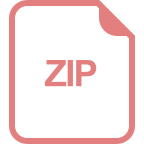