学生表抽象成一个栈,每个学生(包括姓名、学号、成绩)作为栈中的一个元素,该栈采用顺序存储结构,对该栈进行初始化、输出、入栈、出栈和取栈顶元素基本操作,
时间: 2024-01-12 19:05:25 浏览: 28
代码如下:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct {
char name[20];
char id[10];
int score;
} Student;
typedef struct {
Student data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, Student stu) {
if (is_full(s)) {
printf("Stack is full, cannot push!\n");
return;
}
s->top++;
s->data[s->top] = stu;
}
Student pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty, cannot pop!\n");
exit(EXIT_FAILURE);
}
Student stu = s->data[s->top];
s->top--;
return stu;
}
Student top(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty, cannot get top!\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
void print_student(Student stu) {
printf("Name: %s\n", stu.name);
printf("ID: %s\n", stu.id);
printf("Score: %d\n", stu.score);
}
void print_stack(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty!\n");
return;
}
printf("Stack:\n");
for (int i = s->top; i >= 0; i--) {
print_student(s->data[i]);
printf("\n");
}
}
int main() {
Stack s;
init(&s);
Student stu1 = {"Alice", "20210001", 90};
push(&s, stu1);
Student stu2 = {"Bob", "20210002", 85};
push(&s, stu2);
Student stu3 = {"Charlie", "20210003", 95};
push(&s, stu3);
print_stack(&s);
Student top_stu = top(&s);
printf("Top student:\n");
print_student(top_stu);
printf("\n");
Student pop_stu = pop(&s);
printf("Pop student:\n");
print_student(pop_stu);
printf("\n");
print_stack(&s);
return 0;
}
相关推荐
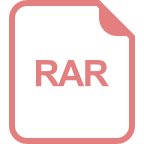
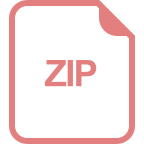













