opencv c++计算轮廓直径的代码
时间: 2023-08-31 21:30:38 浏览: 95
### 回答1:
您好,以下是计算轮廓直径的 OpenCV C 代码示例:
```c
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
Mat image = imread("image.jpg", IMREAD_GRAYSCALE);
if (image.empty())
{
cout << "Could not open or find the image" << endl;
return -1;
}
// Find contours
vector<vector<Point>> contours;
findContours(image, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// Find the largest contour
int maxContourIndex = ;
double maxContourArea = ;
for (int i = ; i < contours.size(); i++)
{
double contourArea = contourArea(contours[i]);
if (contourArea > maxContourArea)
{
maxContourIndex = i;
maxContourArea = contourArea;
}
}
// Calculate the diameter of the largest contour
RotatedRect boundingBox = minAreaRect(contours[maxContourIndex]);
double diameter = max(boundingBox.size.width, boundingBox.size.height);
cout << "The diameter of the largest contour is: " << diameter << endl;
return ;
}
```
希望对您有所帮助。
### 回答2:
计算轮廓直径的代码主要步骤如下:
1. 导入OpenCV库文件,并引用相应的命名空间。
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
```
2. 读取图像,并将其转换为灰度图像。
```cpp
Mat image = imread("input.jpg");
cvtColor(image, image, COLOR_BGR2GRAY);
```
3. 对图像进行二值化处理。
```cpp
threshold(image, image, 127, 255, THRESH_BINARY);
```
4. 查找轮廓。
```cpp
vector<vector<Point>> contours;
vector<Vec4i> hierarchy;
findContours(image, contours, hierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
```
5. 计算每个轮廓的直径,并找到最大直径。
```cpp
double maxDiameter = 0;
for (int i = 0; i < contours.size(); i++) {
double diameter = sqrt(contourArea(contours[i]) / CV_PI) * 2;
if (diameter > maxDiameter) {
maxDiameter = diameter;
}
}
```
6. 输出最大直径。
```cpp
cout << "轮廓直径:" << maxDiameter << endl;
```
完整代码如下:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("input.jpg");
cvtColor(image, image, COLOR_BGR2GRAY);
threshold(image, image, 127, 255, THRESH_BINARY);
vector<vector<Point>> contours;
vector<Vec4i> hierarchy;
findContours(image, contours, hierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
double maxDiameter = 0;
for (int i = 0; i < contours.size(); i++) {
double diameter = sqrt(contourArea(contours[i]) / CV_PI) * 2;
if (diameter > maxDiameter) {
maxDiameter = diameter;
}
}
cout << "轮廓直径:" << maxDiameter << endl;
return 0;
}
```
请注意,以上代码仅为伪代码示例,实际应用中可能需要对图像进行预处理、参数调整等操作,以获取更准确的轮廓直径结果。具体情况需根据实际需求进行相应的修改和优化。
### 回答3:
计算轮廓直径的代码主要分为以下几个步骤:
1. 导入opencv库和其他必要的库文件。
```c
#include <opencv2/opencv.hpp>
#include <iostream>
```
2. 读取图像并转为灰度图像。
```c
cv::Mat src = cv::imread("input.jpg");
cv::Mat gray;
cv::cvtColor(src, gray, cv::COLOR_BGR2GRAY);
```
3. 进行二值化处理。
```c
cv::Mat binary;
cv::threshold(gray, binary, 0, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
```
4. 提取轮廓。
```c
std::vector<std::vector<cv::Point>> contours;
cv::findContours(binary, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
```
5. 计算每个轮廓的直径。
```c
for (int i = 0; i < contours.size(); i++) {
cv::RotatedRect rect = cv::minAreaRect(contours[i]);
float diameter = std::max(rect.size.width, rect.size.height);
std::cout << "Contour " << i + 1 << " diameter: " << diameter << std::endl;
}
```
6. 显示结果。
```c
cv::imshow("Source Image", src);
cv::imshow("Binary Image", binary);
cv::waitKey(0);
```
以上是使用opencv c计算轮廓直径的基本代码。其中,要注意图像读取的路径、二值化方法的选择,以及输出结果的形式等。根据实际需求,可以对代码进行进一步的扩展和优化。
相关推荐
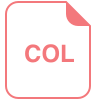
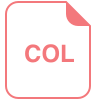
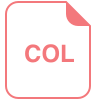
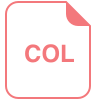
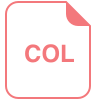









