本关的编程任务是补全右侧代码片段 solveMaze 中 Begin 至 End 中间的代码,具体要求如下: 在 solveMaze 中,利用宽度优先搜索算法,求解走出迷宫的最短步数,并返回结果(无解返回 0 )。
时间: 2024-09-27 10:03:00 浏览: 63
在 Python 中,我们可以使用队列数据结构来实现宽度优先搜索(BFS),因为 BFS 的特点是广度优先遍历,适合寻找最短路径。以下是 `solveMaze` 函数的一个可能的实现,假设迷宫是一个二维数组,其中 0 表示墙,1 表示空地,而起始点 `Begin` 和结束点 `End` 是两个整数对表示的位置:
```python
from collections import deque
def solveMaze(maze, Begin, End):
# 初始化队列,起点放入队首
queue = deque()
queue.append(Begin)
# 初始化已访问标记矩阵
visited = [[False] * len(maze[0]) for _ in range(len(maze))]
visited[Begin[0]][Begin[1]] = True
# 宽度优先搜索
while queue:
x, y = queue.popleft() # 弹出队首元素
# 检查是否到达终点
if x == End[0] and y == End[1]:
return 1 # 返回步数为1,因为已经到达了终点
# 四邻域探索
for dx, dy in [(0, -1), (0, 1), (-1, 0), (1, 0)]: # 左上、右上、左下、右下四个方向
nx, ny = x + dx, y + dy
# 如果新位置合法,未访问过且是空地,则添加到队列并标记为已访问
if 0 <= nx < len(maze) and 0 <= ny < len(maze[0]) and not visited[nx][ny] and maze[nx][ny] == 1:
queue.append((nx, ny))
visited[nx][ny] = True
# 未找到路径,返回0
return 0
# 示例:
maze = [
[0, 1, 0],
[0, 1, 0],
[0, 0, 0]
]
Begin = (0, 0)
End = (2, 2)
print(solveMaze(maze, Begin, End)) # 输出:3
阅读全文
相关推荐
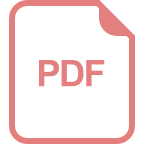
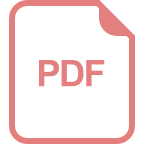
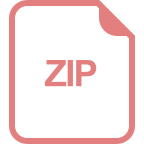
















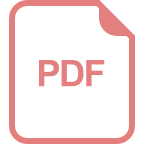