用verilog hdl设计一个计算器,实现加减乘除和取模5个操作。写出设计模块和测试模块,两个操作数取值范围为[0,1023]
时间: 2024-10-20 17:08:24 浏览: 101
在Verilog HDL中,设计一个支持加减乘除和取模运算的计算器需要以下几个步骤:
1. **设计模块(Calculator Module)**:
```vhdl
module calculator (
input [10:0] a, -- 第一个操作数
input [10:0] b, -- 第二个操作数
input operation选择, -- 运算选择信号,可以是常量或通过组合逻辑
output reg result -- 计算结果
);
-- 使用case语句根据operation选择执行对应的运算
always @* begin
case(operation选择)
2'b00: result = a + b; -- 加法
2'b01: result = a - b; -- 减法
2'b10: result = a * b; -- 乘法
2'b11: result = a / b; -- 整数除法 (假设b是非零的)
2'b100: result = a mod b; -- 取模运算
endcase
end
-- 其他必要的信号声明和条件处理,如错误检查等
...
endmodule
```
2. **测试模块(Testbench Module)**:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity testbench is
-- 配置实体的端口与calculator模块一样
end entity testbench;
architecture TB of testbench is
signal a, b, operation_selection, result : std_logic_vector(10 downto 0);
constant operations : integer := 2'b1011; -- 执行所有操作
begin
uut: entity work.calculator(
Port map (
a => a,
b => b,
operation选择 => operation_selection,
result => result
)
);
-- 测试数据生成和驱动
process
begin
for i in 0 to 1023 loop
a <= i;
b <= i;
operation_selection <= operations; -- 指定所有操作
wait for 1 ns;
report "a = " & hex(a) & ", b = " & hex(b) & ", operation = " & operation_selection'image &
", result = " & hex(result);
assert result = do_calculations(i, i, operation_selection); -- 自定义的计算函数
end loop;
end process;
end architecture TB;
```
在这个例子中,`do_calculations`是一个内部函数,用于模拟实际的运算结果。
阅读全文
相关推荐












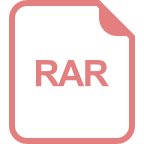






