retrofit协程的取消
时间: 2023-09-18 13:14:00 浏览: 189
在使用 Retrofit 进行网络请求时,如果要支持协程的取消操作,可以使用 Retrofit 的 `suspendCancellableCoroutine` 方法。具体步骤如下:
1. 在 Retrofit 的接口方法中,将返回值类型改为 `Response`。
2. 在接口方法中,通过 `withContext(Dispatchers.IO)` 来切换到 IO 线程进行网络请求。
3. 使用 `suspendCancellableCoroutine` 函数来创建一个协程,将网络请求的结果作为回调。
4. 在协程中,可以通过 `isActive` 来检测协程是否已经被取消,如果已经取消,则可以中断网络请求。
以下是示例代码:
```kotlin
interface ApiService {
@GET("api/data/{category}/{count}/{page}")
suspend fun getData(
@Path("category") category: String,
@Path("count") count: Int,
@Path("page") page: Int
): Response<ResponseBody>
}
suspend fun getData(apiService: ApiService, category: String, count: Int, page: Int): String? {
return suspendCancellableCoroutine { continuation ->
val call = apiService.getData(category, count, page)
continuation.invokeOnCancellation {
call.cancel()
}
call.enqueue(object : Callback<ResponseBody> {
override fun onResponse(call: Call<ResponseBody>, response: Response<ResponseBody>) {
if (response.isSuccessful) {
response.body()?.let {
continuation.resume(it.string())
} ?: run {
continuation.resume(null)
}
} else {
continuation.resume(null)
}
}
override fun onFailure(call: Call<ResponseBody>, t: Throwable) {
if (continuation.isActive) {
continuation.resume(null)
}
}
})
}
}
```
在使用时,可以通过 `CoroutineScope.launch` 来启动一个协程,并在需要取消协程时,调用 `cancel` 函数即可。
```kotlin
val apiService = Retrofit.Builder().baseUrl("https://gank.io/").build().create(ApiService::class.java)
val job = CoroutineScope(Dispatchers.Main).launch {
val result = withContext(Dispatchers.IO) {
getData(apiService, "Android", 10, 1)
}
// 处理网络请求结果
}
job.cancel()
```
阅读全文
相关推荐
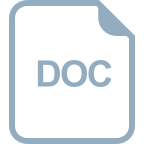
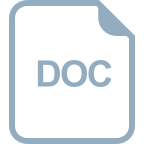
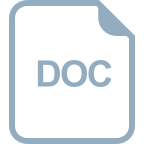
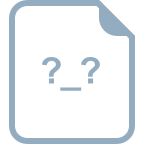
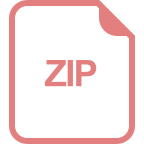
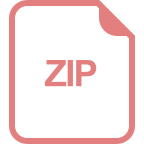
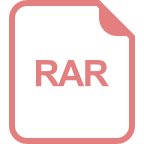
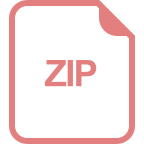
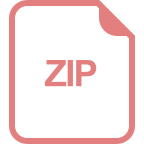
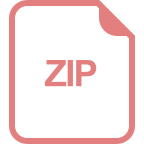
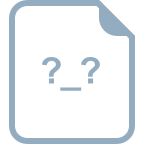
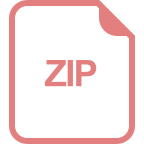
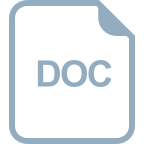
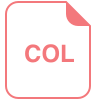
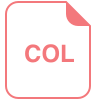
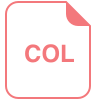


