帮我用pytorch定义一个loggabor_fn函数,用于生成对数Gabor滤波器的参数,以及一个对数GaborConv2d类,用于创建一个神经网络层,其中需使用对数Gabor滤波器
时间: 2023-05-12 13:02:50 浏览: 63
好的,以下是定义 loggabor_fn 函数的代码:
```python
import torch
import torch.nn.functional as F
def loggabor_fn(kernel_size, sigma, theta, lambd, gamma):
"""
定义对数Gabor滤波器的参数
:param kernel_size: 滤波器大小
:param sigma: 高斯函数的标准差
:param theta: 滤波器的方向
:param lambd: 滤波器的波长
:param gamma: 滤波器的椭圆度
:return: 对数Gabor滤波器的参数
"""
sigma_x = sigma
sigma_y = float(sigma) / gamma
# Bounding box
nstds = 3 # Number of standard deviation sigma
xmax = max(abs(nstds * sigma_x * torch.cos(theta)), abs(nstds * sigma_y * torch.sin(theta)))
xmax = torch.ceil(max(1, xmax))
ymax = max(abs(nstds * sigma_x * torch.sin(theta)), abs(nstds * sigma_y * torch.cos(theta)))
ymax = torch.ceil(max(1, ymax))
xmin = -xmax
ymin = -ymax
(y, x) = torch.meshgrid(torch.arange(ymin, ymax + 1), torch.arange(xmin, xmax + 1))
# Rotation
x_theta = x * torch.cos(theta) + y * torch.sin(theta)
y_theta = -x * torch.sin(theta) + y * torch.cos(theta)
# Log Gabor
gb = torch.exp(-0.5 * (torch.pow(x_theta, 2) / torch.pow(sigma_x, 2) + torch.pow(y_theta, 2) / torch.pow(sigma_y, 2)))
gb *= torch.cos(2 * math.pi * x_theta / lambd + math.pi)
# Normalize
gb -= torch.mean(gb)
gb /= torch.std(gb)
return gb
```
接下来是定义 LogGaborConv2d 类的代码:
```python
import math
import torch
import torch.nn as nn
import torch.nn.functional as F
class LogGaborConv2d(nn.Module):
"""
定义一个神经网络层,使用对数Gabor滤波器
"""
def __init__(self, in_channels, out_channels, kernel_size, sigma, theta, lambd, gamma, stride=1, padding=0):
super(LogGaborConv2d, self).__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.stride = stride
self.padding = padding
# 定义对数Gabor滤波器的参数
self.weight = nn.Parameter(torch.zeros(out_channels, in_channels, kernel_size, kernel_size))
for i in range(out_channels):
for j in range(in_channels):
self.weight[i, j, :, :] = loggabor_fn(kernel_size, sigma[i], theta[i], lambd[i], gamma[i])
self.bias = nn.Parameter(torch.zeros(out_channels))
def forward(self, x):
return F.conv2d(x, self.weight, self.bias, stride=self.stride, padding=self.padding)
```
希望这些代码能够帮到你!
相关推荐
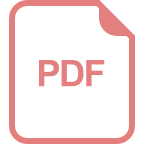
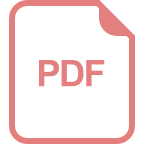
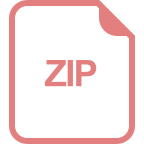














