#include <stdio.h> #include <stdlib.h> struct telnote { int no; char name[20]; char tel[20]; }; int main(void) { int code, i, cnt = 0; char scode[10]; FILE *fp; struct telnote mytel[10], buff, * p; p = &buff; while (1) { printf("\nコード入力:"); gets_s(scode); code = atoi(scode); if (code <= 0)break; p->no = code; printf("氏名入力:"); gets_s(p->name); printf("電話番号入力:"); gets_s(p->tel); mytel[cnt] =buff ; ++cnt; } if (cnt > 0) { fp = fopen("mytel.data", "w"); if (fp == NULL) { printf("file open error!!\n"); exit(1); } printf("\nコード氏名 電話番号\n"); for (i = 0; i < cnt; i++) { printf("%5d %-15s %-15s\n", buff.no, buff.name, buff.tel); fprintf(fp, "%d %s %s\n", mytel[i].no, mytel[i].name, mytel[i].tel); } fclose(fp); } else { printf("\nデータが入力されていません\n"); } return 0; }使代码可以正常运行
时间: 2024-03-29 07:35:49 浏览: 17
首先需要将第一行和第二行之间加上一个空行,否则编译器会将它们当成一个行。
然后,需要将`gets_s`替换为`fgets`,因为`gets_s`在某些编译器上可能会报错,而且`gets_s`不安全,可能导致缓冲区溢出。
最后,需要将`mytel[cnt] = buff;`改为`mytel[cnt] = *p;`,因为`buff`的值在每次输入后都会被覆盖,但是`mytel`数组中存储的是每次输入的值,不能被覆盖。
修改后的代码如下:
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#include<stdio.h>#include<stdlib.h>#include<string.h>
在C语言中,`#include<stdio.h>`、`#include<stdlib.h>`和`#include<string.h>`分别包含了输入/输出、内存管理以及字符串处理相关的库。这里给你演示如何使用这些头文件中的函数:
1. **输入/输出(stdio.h)**:
```c
#include<stdio.h>
int main() {
printf("Hello, World!\n"); // 使用printf输出字符串
scanf("%s", "Enter your name: "); // 使用scanf从用户那里读取输入
return 0;
}
```
2. **内存管理(stdlib.h)**:
```c
#include<stdlib.h>
int *createArray(int size) {
int *array = malloc(size * sizeof(int)); // 分配动态内存
return array;
}
void freeMemory(int *array, int size) {
free(array); // 释放已分配的内存
}
```
3. **字符串处理(string.h)**:
```c
#include<string.h>
char* copyString(const char* src) {
char* dest = (char*)malloc(strlen(src) + 1); // 动态分配内存复制字符串
strcpy(dest, src);
return dest;
}
```
注意,C语言的标准库函数可能需要链接到适当的库,如`-lstdc++` 或 `-lm`,具体取决于编译器设置。
相关推荐
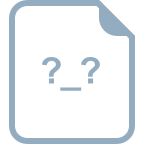
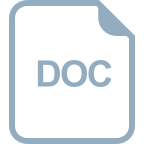
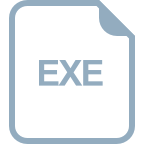













