ubuntu vscode 无法imshow
时间: 2023-09-19 12:07:13 浏览: 207
如果你在使用 Ubuntu 上的 VS Code 编辑器时无法使用 `imshow` 显示图像,可能是由于缺少适当的图形环境或配置不正确导致的。VS Code 默认情况下不支持直接显示图像。
要在 VS Code 中显示图像,可以尝试以下方法:
1. 使用 Matplotlib 库:如果你使用的是 Python 并且想要在 VS Code 中显示图像,可以使用 Matplotlib 库。在你的代码中添加以下行:
```python
import matplotlib.pyplot as plt
# 显示图像
plt.imshow(image)
plt.show()
```
这将使用 Matplotlib 的图形界面显示图像。
2. 使用 Jupyter 扩展:如果你在 VS Code 中使用 Jupyter 扩展,可以使用 Jupyter Notebook 功能来显示图像。创建一个新的 Jupyter Notebook 文件,将图像代码复制到该文件中,并运行代码块以显示图像。
3. 使用其他图像查看器:在 VS Code 中,你可以使用其他外部图像查看器来打开图像文件。右键单击图像文件,选择 "Open With"(使用以下方式打开),然后选择你喜欢的图像查看器。
请注意,以上方法适用于在 VS Code 中显示图像,但需要适当的图形环境和依赖项支持。确保你已经安装了所需的图像处理库和依赖项,并且配置正确。
如果问题仍然存在,请提供更多详细信息,例如代码示例、错误消息或配置信息,以便我能够更好地帮助你解决问题。
相关问题
ubuntu vscode 配置c++ opencv环境
### 配置 Ubuntu 上 VSCode 的 C++ 和 OpenCV 开发环境
#### 安装必要的依赖项
为了确保开发环境顺利搭建,在开始之前需确认已安装所有必需的软件包。可以通过以下命令来更新并安装这些工具:
```bash
sudo apt update && sudo apt upgrade -y
sudo apt install build-essential cmake git pkg-config libgtk-3-dev \
libavcodec-dev libavformat-dev libswscale-dev python3-dev python3-numpy \
libtbb2 libtbb-dev libjpeg-dev libpng-dev libtiff-dev gfortran openexr \
libatlas-base-dev
```
此操作会准备系统以支持后续的编译工作[^1]。
#### 下载并构建 OpenCV
获取指定版本的 OpenCV 源码,并按照如下方式完成其本地化部署:
```bash
cd ~
git clone https://github.com/opencv/opencv.git
cd opencv
mkdir build && cd build
cmake ..
make -j$(nproc)
sudo make install
```
上述过程将从 GitHub 获取最新的源代码,创建一个用于编译的临时文件夹 `build` 并通过 CMake 工具链进行配置,最后利用多线程加速编译流程以及安装最终产物到默认位置[^2]。
#### 设置 VSCode 支持 C++
安装 Microsoft 提供的官方扩展插件 “C/C++”,它提供了 IntelliSense 功能以及其他特性帮助编写高效的 C++ 代码;同时还需要设置好 launch.json 文件以便调试程序:
```json
{
"version": "0.2.0",
"configurations": [
{
"name": "(gdb) Launch",
"type": "cppdbg",
"request": "launch",
"program": "${workspaceFolder}/your_program_name",
"args": [],
"stopAtEntry": false,
"cwd": "${fileDirname}",
"environment": [],
"externalConsole": true,
"MIMode": "gdb",
"setupCommands": [
{
"description": "Enable pretty-printing for gdb",
"text": "-enable-pretty-printing",
"ignoreFailures": true
}
],
"preLaunchTask": "compile"
}
]
}
```
这段 JSON 片段定义了一个简单的启动配置,其中包含了指向可执行文件的位置和其他选项,使得开发者可以方便地测试自己的应用程序[^3]。
#### 创建任务用于自动化编译
编辑 `.vscode/tasks.json` 来简化日常工作中频繁使用的编译指令集:
```json
{
"tasks": [
{
"label": "compile",
"command": "/usr/bin/cmake",
"args": ["--build", "."],
"group": {
"kind": "build",
"isDefault": true
},
"problemMatcher": []
}
]
}
```
这里指定了一个名为 compile 的任务,当按下 Ctrl+Shift+B 组合键时自动触发该任务执行一次完整的重新编译动作。
#### 测试环境有效性
编写一段简单的小例子验证当前环境是否能够正常运作,比如读取图像显示窗口等基本功能:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
int main(int argc, char **argv){
cv::Mat image;
image = cv::imread("/path/to/image.jpg");
if (image.empty()){
std::cout << "Could not read the image." << std::endl ;
return 1;
}
cv::imshow("Display window", image);
int k = cv::waitKey(0); // Wait infinitely until a keypress event occurs
return 0;
}
```
保存以上代码片段至合适路径下作为入口点,随后尝试运行看看能否成功加载图片资源并弹出预览界面。
ubuntu vscode 下 opencv怎么加载图片并显示
在Ubuntu系统中使用VSCode结合OpenCV加载并显示图片的步骤如下:
1. 确保你的Ubuntu系统中已经安装了OpenCV库。如果未安装,可以通过终端使用包管理器进行安装,例如使用`sudo apt-get install libopencv-dev`。
2. 安装VSCode,如果已经安装,确保安装了C++和Python的扩展,以便于编写和运行代码。
3. 打开VSCode,创建一个新的C++文件,比如命名为`main.cpp`。
4. 编写代码,以下是一个简单的示例代码,展示如何使用OpenCV在Ubuntu下加载并显示图片:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
int main() {
// 图片路径,请根据实际情况进行修改
cv::String image_path = "/path/to/your/image.jpg";
// 使用cv::imread函数读取图片
cv::Mat image = cv::imread(image_path, cv::IMREAD_COLOR);
if(image.empty()) {
std::cout << "图片加载失败" << std::endl;
return -1;
}
// 使用cv::imshow函数显示图片
cv::imshow("显示窗口", image);
// 等待按键,设置等待时间为任意正数,这里设置为0表示无限等待
cv::waitKey(0);
return 0;
}
```
5. 确保你的编译器配置正确,并且能够在终端中使用g++编译器。在VSCode中,你可以通过运行配置或任务来指定编译器和编译参数。
6. 编译代码,如果一切配置正确,你应该能够编译出可执行文件。
7. 运行编译出的程序,如果图片路径正确,你将看到一个窗口显示了加载的图片。
8. 在图片窗口中点击任意键,程序将结束并关闭窗口。
阅读全文
相关推荐
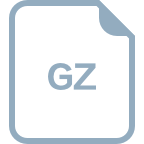














