subprocess.run方法
时间: 2023-06-04 12:07:42 浏览: 195
`subprocess.run` 是 Python 中用于在程序中运行其他程序的方法。它是 Python 3.5 版本引入的,可以用来替代旧版本中的 `subprocess.call` 和 `subprocess.check_call` 等函数。
使用 `subprocess.run` 方法,你可以很方便地在 Python 中调用其他命令行程序,并且能够简单地获取命令的输出结果。此外,该方法还支持设置超时、设置环境变量等高级功能。
举个例子:
```
import subprocess
result = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE)
print(result.stdout.decode())
```
上面的代码会在 Python 中运行 `ls -l` 命令,并将输出结果存储在 `result` 变量中。
相关问题
subprocess.run方法之后程序输出的结果呢?
### 回答1:
subprocess.run方法返回一个CompletedProcess对象,其中包含了执行命令的结果,可以通过访问其属性来获取输出结果。例如:
```python
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
```
这个例子中,我们执行了ls -l命令,使用了capture_output=True选项来捕获输出,text=True选项来将输出以字符串的形式返回。然后我们通过访问result.stdout属性来获取命令输出的结果。
### 回答2:
subprocess.run方法执行后,程序会根据指定的命令运行一个子进程,并等待子进程执行结束。执行结束后,subprocess.run方法会返回一个CompletedProcess对象,该对象包含了一些有关子进程执行结果的信息。
CompletedProcess对象有以下几个属性可以获取结果信息:
- args:保存传递给subprocess.run方法的命令参数。
- returncode:保存子进程的返回码,通常用于判断子进程是否执行成功。一般来说,返回码为0表示执行成功,非0表示执行失败。
- stdout:保存子进程的标准输出内容。如果在调用subprocess.run时设置了capture_output参数为True,则可以通过该属性获取标准输出内容。
- stderr:保存子进程的标准错误输出内容。如果在调用subprocess.run时设置了capture_output参数为True,则可以通过该属性获取标准错误输出内容。
要获取这些结果信息,可以通过调用CompletedProcess对象的相应属性来获取。例如,可以使用completed.stdout来获取标准输出内容,使用completed.returncode来获取返回码。
另外,还可以通过判断子进程的返回码来确定子进程是否执行成功。如果返回码为0,则说明子进程执行成功;如果返回码为非0,则说明子进程执行失败。
总而言之,subprocess.run方法执行后,可以通过CompletedProcess对象获取子进程执行的结果信息,包括返回码、标准输出等,并据此判断子进程是否执行成功。
### 回答3:
subprocess.run方法是Python中用于运行外部命令的函数。当我们调用subprocess.run方法后,程序会执行指定的外部命令,并且返回一个CompletedProcess对象。
CompletedProcess对象包含了外部命令的执行结果相关的信息,其中最重要的属性是returncode。如果returncode的值为0,表示外部命令顺利执行完毕;如果returncode的值不为0,表示外部命令执行出错。
除了returncode属性,CompletedProcess对象还包含了其他一些属性,如args表示执行的外部命令,stdout表示命令的标准输出,stderr表示命令的标准错误输出等。
如果我们需要获取程序的输出结果,可以通过CompletedProcess对象的stdout属性来获取。stdout属性是一个字节流(bytes),我们可以使用.decode("UTF-8")将其转换为字符串形式。
总结起来,subprocess.run方法执行外部命令后,程序输出的结果是一个CompletedProcess对象,我们可以通过该对象的returncode属性来判断命令执行结果是否正确,而stdout属性可以用来获取命令的输出结果。
subprocess.Popen subprocess.run
subprocess.Popen and subprocess.run are both modules in Python's subprocess module that are used for running external commands. Here's a brief explanation of each:
1. subprocess.Popen: This function is used to create a new process and execute a command within it. It provides more flexibility and control over the execution process. Popen returns a Popen object, which allows you to interact with the running process, such as reading its output or terminating it.
Example usage:
```python
import subprocess
# Run a command and get its output
result = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE)
output = result.communicate()
print(output.decode('utf-8'))
```
2. subprocess.run: This function is a higher-level convenience function introduced in Python 3.5. It is used to run a command and wait for it to complete. It returns a CompletedProcess object that provides information about the completed process, such as return code and output.
Example usage:
```python
import subprocess
# Run a command and get its output
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
```
Both functions can be used to execute external commands, but the choice between them depends on your requirements. Popen offers more flexibility, while run provides a simpler and more straightforward way to execute commands.
阅读全文
相关推荐
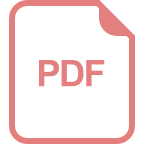
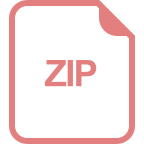
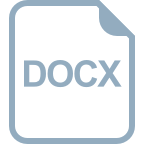







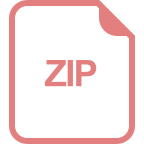
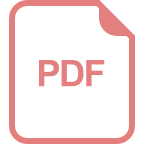
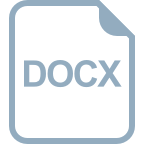
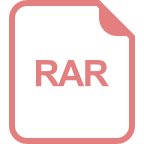
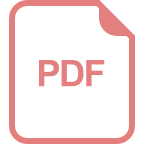