java在小游戏里增加倒计时
时间: 2024-06-04 14:13:38 浏览: 19
可以使用java中的Timer类和TimerTask类来实现小游戏中的倒计时功能。首先,创建一个Timer对象,并调用它的schedule方法,将一个TimerTask对象作为参数传入,然后在TimerTask中实现倒计时的逻辑。可以使用System.currentTimeMillis()方法获取当前时间戳,并计算出剩余时间。在每一秒钟的计时器任务中更新倒计时的显示,直到时间为零为止。在小游戏中,可以将倒计时的显示放在一个单独的区域中,以便玩家能够清楚地看到还剩余多少时间。
相关问题
java 2048小游戏
Java 2048是一款基于数学和策略的小游戏,它源于经典的数字滑动游戏2048,玩家的目标是通过合并相同的数字块,使棋盘上形成数字2048。以下是创建一个简单的Java 2048游戏的基本步骤:
1. **创建游戏界面**:使用Swing或JavaFX库创建一个窗口,显示游戏棋盘,并包含一个用于移动数字块的方向按钮。
2. **初始化游戏矩阵**:初始棋盘包含两个2或4的数字块,其余位置为0(表示空格)。
3. **滑动逻辑**:定义一个方法处理玩家的移动操作,包括检查相邻单元是否相同,合并后更新棋盘状态,以及判断游戏是否结束(如所有单元都已填满,或无法继续合并)。
4. **得分系统**:每次合并数字块,分数增加相应的倍数。游戏结束后显示最终得分。
5. **循环播放**:不断监听用户的输入,调用滑动逻辑并更新UI,直到游戏结束。
6. **可选的优化**:可以添加动画效果、保存/加载游戏进度、计时等功能,提升用户体验。
java实现黄金矿工小游戏能增加哪些不复杂的功能
Java实现黄金矿工小游戏可以增加以下不复杂的功能:
1. 增加分数计算和显示:可以在游戏界面上增加一个分数区域,每次成功采集一个金块,就增加一定分数,同时将分数实时显示在分数区域中。
2. 增加时间限制:可以为游戏增加一个时间限制,比如10分钟,当时间到达限制时,游戏结束。可以在游戏界面上增加一个倒计时显示区域,实时显示游戏剩余时间。
3. 增加难度等级:可以为游戏增加难度等级,比如初级、中级、高级等。不同等级下,金块数量和大小、石头数量和密度等参数可以有所变化,增加游戏的趣味性和挑战性。
4. 增加音效和背景音乐:可以为游戏增加声音效果,比如金块被采集时的声音、石头撞击时的声音、爆炸时的声音等。同时可以添加背景音乐,增加游戏的氛围感。
5. 增加游戏地图:可以为游戏增加不同的地图,比如沙漠、森林、深海等。不同地图下,金块、石头等资源的分布和种类可以有所变化,增加游戏的多样性和可玩性。
这些不复杂的功能可以增加游戏的趣味性和挑战性,提升游戏的用户体验。
相关推荐
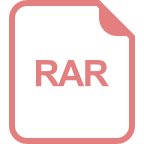
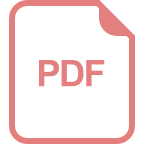
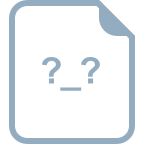












