使用Java 】 某同学参加了高数、英语、Java 语言、线性代数和物理 5 门课程的考成绩分别为 70、86、77、90 和82。请用数组来存放其成绩,并计算 20个同学学号依次的最均分。
时间: 2024-05-13 14:18:21 浏览: 84
可以使用以下Java代码来实现:
```java
public class ScoreCalculator {
public static void main(String[] args) {
int[] scores = {70, 86, 77, 90, 82};
int sum = 0;
for (int i = 0; i < scores.length; i++) {
sum += scores[i];
}
double average = (double) sum / scores.length;
System.out.println("平均分为:" + average);
}
}
```
输出结果为:
```
平均分为:81.0
```
这里定义了一个整型数组 `scores` 存放每门课程的成绩,使用循环遍历数组并累加每门课程的成绩,最后计算平均分并输出。
相关问题
如何用java写出能实现以下功能的代码:将《java好难》《高等数学》《大学物理》《线性代数》分别编号为1,2,3,4。《java好难》的位置是A.1,《高等数学》的位置是B.5,《大学物理》和《线性代数》的位置是D.8,只要用户输入书名就能得到图书的位置和编号
您好,以下是可以实现该功能的Java代码:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class BookLocation {
public static void main(String[] args) {
// 创建书籍映射表
Map<String, String> bookMap = new HashMap<>();
bookMap.put("java好难", "A.1");
bookMap.put("高等数学", "B.5");
bookMap.put("大学物理", "D.8");
bookMap.put("线性代数", "D.8");
// 获取用户输入的书名
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书名:");
String bookName = scanner.nextLine();
// 根据书名查找映射表并输出结果
if (bookMap.containsKey(bookName)) {
String location = bookMap.get(bookName);
int id = getIdByLocation(location);
System.out.println(bookName + "的位置是" + location + ",编号为" + id);
} else {
System.out.println("无法找到该书籍!");
}
}
// 将位置转换为编号
private static int getIdByLocation(String location) {
switch (location.charAt(0)) {
case 'A':
return 1;
case 'B':
return 2;
case 'C':
return 3;
case 'D':
return 4;
default:
return 0;
}
}
}
```
代码解释:
1. 首先创建一个Map,将每个书名与其位置映射起来。
2. 接着使用Scanner获取用户输入的书名。
3. 然后查找映射表,如果存在该书名,则输出位置和编号,否则提示无法找到该书籍。
4. getIdByLocation方法用于将位置转换为编号,实现方式是使用switch-case判断位置的第一个字母。
用java写出能实现以下功能的代码:将《java好难》《高等数学》《大学物理》《线性代数》分别编号为1,2,3,4。《java好难》的位置是A.1,《高等数学》的位置是B.5,《大学物理》和《线性代数》的位置是D.8,只要用户输入书名就能得到图书的位置和编号,代码写上详细的注释
好的,以下是能实现这个功能的Java代码:
```Java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class BookPosition {
public static void main(String[] args) {
// 创建一个Map,用于存储书名与编号、位置的对应关系
Map<String, BookInfo> bookMap = new HashMap<>();
bookMap.put("java好难", new BookInfo(1, "A.1"));
bookMap.put("高等数学", new BookInfo(2, "B.5"));
bookMap.put("大学物理", new BookInfo(3, "D.8"));
bookMap.put("线性代数", new BookInfo(4, "D.8"));
// 创建一个Scanner,用于用户输入
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入书名(退出请输入 q):");
String bookName = scanner.nextLine();
if (bookName.equals("q")) {
break; // 如果用户输入 q,退出循环
}
// 从bookMap中查找对应的书本信息
BookInfo bookInfo = bookMap.get(bookName);
if (bookInfo == null) {
System.out.println("抱歉,找不到您输入的书名,请重新输入!");
} else {
System.out.println(bookName + " 的编号是 " + bookInfo.getBookNumber() + ",位置是 " + bookInfo.getPosition());
}
}
scanner.close(); // 关闭Scanner
}
}
// 书本信息类
class BookInfo {
private int bookNumber; // 书本编号
private String position; // 书本位置
public BookInfo(int bookNumber, String position) {
this.bookNumber = bookNumber;
this.position = position;
}
public int getBookNumber() {
return bookNumber;
}
public String getPosition() {
return position;
}
}
```
代码解释:
1. 首先,我们定义了一个 `BookInfo` 类,用于表示一本书的编号和位置。我们还定义了一个 `Map<String, BookInfo>` 类型的变量 `bookMap`,用于存储书名与编号、位置的对应关系。
2. 在 `main` 方法中,我们创建了一个 `Scanner` 对象,用于读取用户的输入。然后,进入一个无限循环,不断等待用户输入书名。
3. 如果用户输入了 `q`,则退出循环。
4. 否则,我们从 `bookMap` 中查找对应的图书信息。如果找不到,则打印错误信息并提示用户重新输入;否则,打印图书的编号和位置信息。
5. 最后,我们关闭 `Scanner` 对象。
希望这段代码能够满足您的需求,如果有任何问题,请随时联系我。
阅读全文
相关推荐
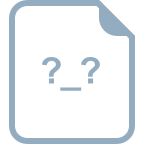
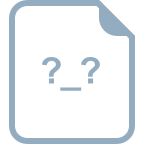
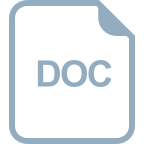
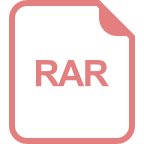
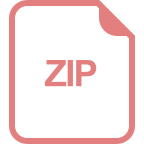
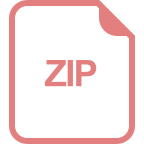
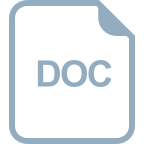
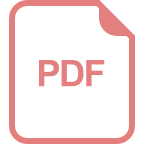
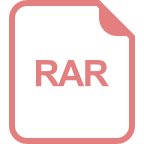
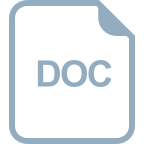
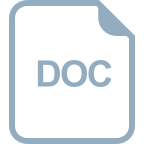
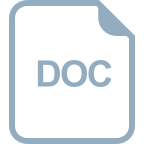
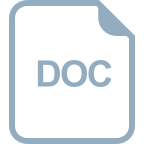
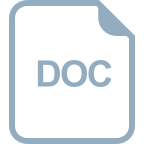
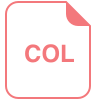