点类point的设计与定义,point成员有x,y。成员函数有构造,set,get,移动,比较。测试point类
时间: 2024-05-01 11:21:09 浏览: 17
以下是点类 Point 的设计与定义:
```cpp
class Point {
private:
int x;
int y;
public:
Point() {
x = 0;
y = 0;
}
Point(int x, int y) {
this->x = x;
this->y = y;
}
void setX(int x) {
this->x = x;
}
void setY(int y) {
this->y = y;
}
int getX() const {
return x;
}
int getY() const {
return y;
}
void move(int offsetX, int offsetY) {
x += offsetX;
y += offsetY;
}
bool operator==(const Point& other) const {
return x == other.x && y == other.y;
}
bool operator!=(const Point& other) const {
return !(*this == other);
}
};
```
在这个点类中,我们定义了两个私有成员变量 x 和 y,表示点在二维坐标系中的位置。为了方便使用,我们提供了两个构造函数,一个默认构造函数和一个带有 x 和 y 参数的构造函数。同时,我们还提供了 set 和 get 函数,用于设置和获取 x 和 y 的值,以及一个 move 函数,用于移动点的位置。最后,我们还重载了 == 和 != 运算符,用于比较两个点是否相等。
接下来是一个简单的测试代码,用于测试 Point 类的功能:
```cpp
int main() {
Point p1; // 创建一个默认点
Point p2(3, 4); // 创建一个坐标为 (3, 4) 的点
cout << "p1: (" << p1.getX() << ", " << p1.getY() << ")" << endl;
cout << "p2: (" << p2.getX() << ", " << p2.getY() << ")" << endl;
p1.setX(1);
p1.setY(2);
cout << "p1: (" << p1.getX() << ", " << p1.getY() << ")" << endl;
p2.move(-1, 2);
cout << "p2: (" << p2.getX() << ", " << p2.getY() << ")" << endl;
if (p1 == p2) {
cout << "p1 equals p2" << endl;
}
else {
cout << "p1 not equals p2" << endl;
}
return 0;
}
```
输出结果如下:
```
p1: (0, 0)
p2: (3, 4)
p1: (1, 2)
p2: (2, 6)
p1 not equals p2
```
可以看到,Point 类的功能正常运行。
相关推荐
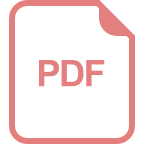
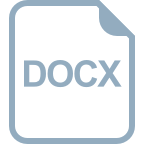












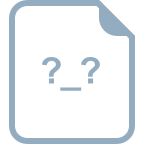
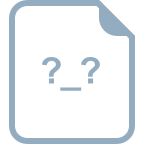
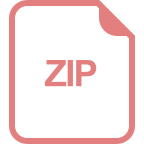