蒙特卡洛交叉验证去除异常样本代码
时间: 2023-08-28 20:04:42 浏览: 591
蒙特卡洛交叉验证(Monte Carlo Cross Validation,简称MCCV)通过随机选择样本数据集的子集来进行交叉验证,从而减少异常样本的影响。以下是使用Python实现MCCV去除异常样本的示例代码:
```python
import numpy as np
from sklearn.model_selection import ShuffleSplit
def mccv(X, y, estimator, n_splits=10, test_size=0.1, random_state=None):
# 进行MCCV
cv = ShuffleSplit(n_splits=n_splits, test_size=test_size, random_state=random_state)
scores = []
for train_index, test_index in cv.split(X):
estimator.fit(X[train_index], y[train_index])
score = estimator.score(X[test_index], y[test_index])
scores.append(score)
# 计算得分的均值和标准差
mean_score = np.mean(scores)
std_score = np.std(scores)
# 计算异常值的上下限
upper_limit = mean_score + 2 * std_score
lower_limit = mean_score - 2 * std_score
# 找到异常样本的索引
outlier_index = np.where((scores > upper_limit) | (scores < lower_limit))[0]
# 去除异常样本
X_clean = np.delete(X, outlier_index, axis=0)
y_clean = np.delete(y, outlier_index, axis=0)
return X_clean, y_clean
```
使用示例:
```python
from sklearn.datasets import load_iris
from sklearn.linear_model import LogisticRegression
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 初始化逻辑回归模型
estimator = LogisticRegression()
# 进行MCCV去除异常样本
X_clean, y_clean = mccv(X, y, estimator)
# 在去除异常样本后的数据集上训练模型
estimator.fit(X_clean, y_clean)
```
在上述示例中,我们使用鸢尾花数据集进行演示。首先,我们通过调用`mccv`函数进行MCCV,并传入数据集、逻辑回归模型以及其他参数。该函数将返回去除异常样本后的`X_clean`和`y_clean`。然后,我们可以在去除异常样本后的数据集上训练模型。
阅读全文
相关推荐
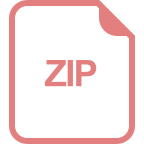
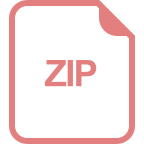
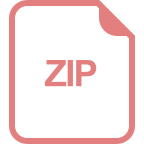
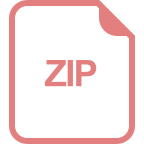
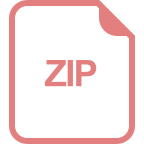
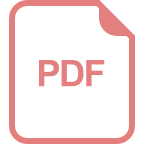
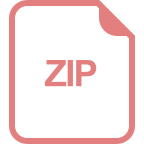
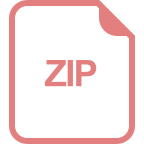
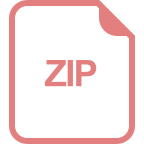
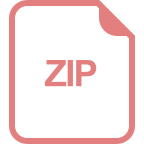
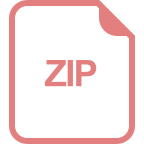
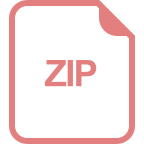