c语言代码实现顺序栈的基本操作
时间: 2023-10-26 08:21:04 浏览: 79
可以实现顺序栈的基本操作的C语言代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100 // 定义栈的大小
typedef int ElemType; // 栈内元素类型
typedef struct {
ElemType data[MAX_SIZE]; // 存储栈内元素的数组
int top; // 栈顶指针
} SqStack; // 定义顺序栈类型
void InitStack(SqStack *S); // 初始化栈
int StackEmpty(SqStack *S); // 判断栈是否为空
int StackFull(SqStack *S); // 判断栈是否已满
void Push(SqStack *S, ElemType x); // 入栈操作
ElemType Pop(SqStack *S); // 出栈操作
ElemType GetTop(SqStack *S); // 获取栈顶元素值
int main() {
SqStack S;
int i;
ElemType x, top;
InitStack(&S); // 初始化栈
// 生成元素
for (i = 1; i <= 10; i++) {
x = i;
Push(&S, x); // 入栈
}
while (!StackEmpty(&S)) {
top = GetTop(&S); // 获取栈顶元素值
printf("%d ", top); // 打印栈顶元素值
Pop(&S); // 出栈
}
return 0;
}
// 初始化栈
void InitStack(SqStack *S) {
S->top = -1;
}
// 判断栈是否为空
int StackEmpty(SqStack *S) {
if (S->top == -1) {
return 1; // 栈为空
}
return 0; // 栈不为空
}
// 判断栈是否已满
int StackFull(SqStack *S) {
if (S->top == MAX_SIZE - 1) {
return 1; // 栈已满
}
return 0; // 栈未满
}
// 入栈操作
void Push(SqStack *S, ElemType x) {
if (StackFull(S)) {
printf("Error: Stack Full.\n"); // 栈已满,无法入栈
return;
}
S->top++; // 栈顶指针加1
S->data[S->top] = x; // 新元素入栈
}
// 出栈操作
ElemType Pop(SqStack *S) {
ElemType x;
if (StackEmpty(S)) {
printf("Error: Stack Empty.\n"); // 栈为空,无法出栈
return -1;
}
x = S->data[S->top]; // 栈顶元素出栈
S->top--; // 栈顶指针减1
return x; // 返回栈顶元素值
}
// 获取栈顶元素值
ElemType GetTop(SqStack *S) {
if (StackEmpty(S)) {
printf("Error: Stack Empty.\n"); // 栈为空,无法获取栈顶元素值
return -1;
}
return S->data[S->top]; // 返回栈顶元素值
}
```
相关推荐
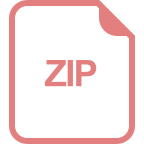
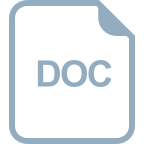














