用C语言实现顺序栈的所有操作
时间: 2024-03-10 16:46:02 浏览: 23
好的,以下是用C语言实现顺序栈的基本操作:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100 // 定义栈的最大容量
typedef struct {
int data[MAXSIZE]; // 栈内元素
int top; // 栈顶指针
} SeqStack;
// 初始化栈
void InitStack(SeqStack *S) {
S->top = -1;
}
// 判断栈是否为空
int IsEmpty(SeqStack S) {
if (S.top == -1) {
return 1;
} else {
return 0;
}
}
// 判断栈是否已满
int IsFull(SeqStack S) {
if (S.top == MAXSIZE - 1) {
return 1;
} else {
return 0;
}
}
// 进栈
int Push(SeqStack *S, int x) {
if (IsFull(*S)) {
return 0;
} else {
S->top++;
S->data[S->top] = x;
return 1;
}
}
// 出栈
int Pop(SeqStack *S, int *x) {
if (IsEmpty(*S)) {
return 0;
} else {
*x = S->data[S->top];
S->top--;
return 1;
}
}
// 获取栈顶元素
int GetTop(SeqStack S, int *x) {
if (IsEmpty(S)) {
return 0;
} else {
*x = S.data[S.top];
return 1;
}
}
int main() {
SeqStack S;
InitStack(&S);
int i;
for (i = 1; i <= 10; i++) {
Push(&S, i);
}
int x;
Pop(&S, &x);
printf("出栈元素为:%d\n", x);
GetTop(S, &x);
printf("栈顶元素为:%d\n", x);
return 0;
}
```
注意:以上代码仅为顺序栈的基本操作,根据实际需求还可以进行其他操作,例如清空栈、销毁栈等。
相关推荐
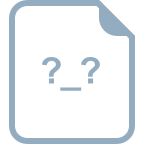
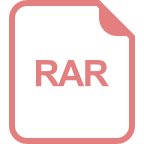














