用c语言做项目要求用到函数,数组,指针,循环,文件
时间: 2024-03-26 09:37:47 浏览: 121
好的,我知道了。你要求用C语言做项目,需要用到函数、数组、指针、循环以及文件操作。这些都是C语言中非常基础和重要的知识点,下面我简单介绍一下。
1. 函数:函数是C语言中的基本模块,它可以将一段代码封装成一个功能,方便代码的复用和维护。在C语言中,函数的定义包括函数名、参数列表和函数体。函数可以返回一个值,也可以不返回任何值。
2. 数组:数组是C语言中常用的数据结构,它可以存储一组相同类型的数据。在C语言中,数组的定义需要指明数组的类型、名称和长度。数组的元素可以通过下标访问,下标从0开始。
3. 指针:指针是C语言中非常重要的概念,它可以指向一个变量的地址。通过指针,可以实现对变量的间接访问和修改。在C语言中,指针的定义需要指明指针的类型和名称。指针可以通过取地址符“&”获取变量的地址,通过间接访问符“*”访问指针指向的变量。
4. 循环:循环是C语言中的一种控制结构,它可以重复执行一段代码。C语言中有三种循环结构:for循环、while循环和do-while循环。for循环适用于已知循环次数的情况,while循环适用于不知道循环次数但有循环条件的情况,do-while循环适用于至少执行一次的情况。
5. 文件操作:文件操作是C语言中用于读写文件的函数。在C语言中,文件操作需要打开文件、读取或写入数据、关闭文件。文件操作可以使用标准库中的函数,如fopen、fread、fwrite、fclose等。
希望以上内容能够帮助你更好地理解和使用C语言。
相关问题
用C语言做一个用到数组、函数、指针、循环结构、分支结构的旅游小程序
好的,以下是一个简单的旅游小程序代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体表示旅游景点信息
typedef struct {
char name[50];
char location[50];
int rating;
int price;
} Destination;
// 定义函数获取用户输入
void get_input(char *prompt, char *buffer, int buffer_size) {
printf("%s", prompt);
fgets(buffer, buffer_size, stdin);
// 去掉末尾的换行符
buffer[strcspn(buffer, "\n")] = 0;
}
// 定义函数打印景点信息
void print_destination(Destination *dest) {
printf("%s (%s)\n", dest->name, dest->location);
printf("Rating: %d stars\n", dest->rating);
printf("Price: $%d\n", dest->price);
}
// 定义函数查找景点
Destination *find_destination(Destination *destinations, int num_destinations, char *name) {
for (int i = 0; i < num_destinations; i++) {
if (strcmp(destinations[i].name, name) == 0) {
return &destinations[i];
}
}
return NULL;
}
// 主函数
int main() {
Destination destinations[] = {
{"Great Wall", "China", 5, 20},
{"Eiffel Tower", "France", 4, 25},
{"Statue of Liberty", "USA", 3, 30},
{"Pyramids", "Egypt", 4, 15}
};
int num_destinations = sizeof(destinations) / sizeof(Destination);
// 打印所有景点信息
printf("Available destinations:\n");
for (int i = 0; i < num_destinations; i++) {
print_destination(&destinations[i]);
}
char name[50];
get_input("\nEnter destination name: ", name, sizeof(name));
// 查找用户输入的景点
Destination *dest = find_destination(destinations, num_destinations, name);
if (dest == NULL) {
printf("Destination not found.\n");
return 0;
}
// 打印用户选择的景点信息
printf("\nYou selected:\n");
print_destination(dest);
// 定义指针变量并分配内存空间
int *num_tickets = malloc(sizeof(int));
if (num_tickets == NULL) {
printf("Memory allocation failed.\n");
return 0;
}
// 获取用户输入的门票数量
get_input("Enter number of tickets: ", name, sizeof(name));
*num_tickets = atoi(name);
// 根据门票数量计算总价
int total_price = *num_tickets * dest->price;
printf("\nTotal price: $%d\n", total_price);
// 释放内存空间
free(num_tickets);
return 0;
}
```
该程序使用了数组来存储旅游景点信息,使用函数来获取用户输入、打印景点信息、查找景点等操作,使用指针来动态分配内存空间并在程序结束时释放。程序中还使用了循环结构和分支结构来实现不同的功能。
用C语言编写一个程序要求用到函数int find(int *p,int n,int x),在指针p所指的数组中查找整型数x,如果x在数组中,则该函数返回1,否则返回0。n为数组的大小。
好的,我来帮您编写这个程序:
```
#include <stdio.h>
int find(int *p, int n, int x);
int main()
{
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 3;
int result = find(arr, n, x);
if (result == 1)
{
printf("%d is found in the array.\n", x);
}
else
{
printf("%d is not found in the array.\n", x);
}
return 0;
}
int find(int *p, int n, int x)
{
for (int i = 0; i < n; i++)
{
if (*(p + i) == x)
{
return 1;
}
}
return 0;
}
```
在这个程序中,我们定义了一个`find`函数来查找整型数x是否在指针p所指的数组中。在`main`函数中,我们定义了一个整型数组`arr`,数组大小为`n`,要查找的整数为`x`。调用`find`函数来判断`x`是否在`arr`数组中,并根据结果输出相应的信息。
在`find`函数中,我们使用了一个`for`循环来遍历数组中的每一个元素。如果找到了`x`,则返回1,否则返回0。在数组中查找元素需要注意的是,我们使用指针来访问数组元素,即使用`*(p + i)`来代替`p[i]`,这两种写法是等价的。
阅读全文
相关推荐
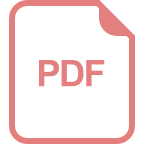
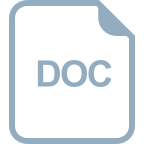
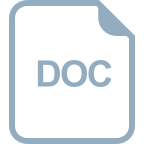
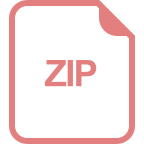
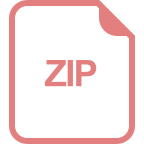
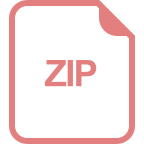
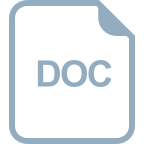
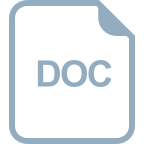
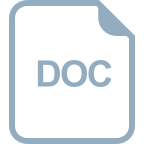
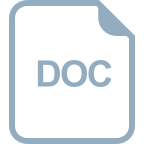
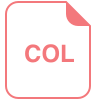


