如何使用树莓派C语言控制ws2812灯带
时间: 2024-02-21 16:24:10 浏览: 29
控制ws2812灯带,需要用到树莓派上的GPIO口,以及相关的C语言库。以下是一个简单的操作流程:
1. 连接ws2812灯带到树莓派上,可以使用杜邦线将灯带的DI引脚连接到树莓派的GPIO口(例如GPIO18)上。
2. 安装控制ws2812灯带的C语言库,例如rpi_ws281x。可以先从Github上下载rpi_ws281x库的源代码,然后编译和安装。具体步骤如下:
```shell
# 从Github上下载rpi_ws281x库的源代码
git clone https://github.com/jgarff/rpi_ws281x.git
# 进入rpi_ws281x目录
cd rpi_ws281x
# 编译和安装库
sudo apt-get install scons
scons
cd python
sudo python setup.py install
```
3. 编写控制ws2812灯带的C语言程序,使用rpi_ws281x库提供的函数进行控制。例如:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <unistd.h>
#include <string.h>
#include <signal.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/types.h>
#include <sys/stat.h>
#include "ws2811.h"
#define TARGET_FREQ WS2811_TARGET_FREQ
#define GPIO_PIN 18
#define DMA 5
int main()
{
int i;
ws2811_t ledstring =
{
.freq = TARGET_FREQ,
.dmanum = DMA,
.channel =
{
[0] =
{
.gpionum = GPIO_PIN,
.count = 8,
.invert = 0,
.brightness = 255,
},
},
};
if(ws2811_init(&ledstring))
{
printf("ws2811_init failed\n");
return -1;
}
// Define the colors of the LED pixels.
ws2811_led_t colors[3] =
{
0x00ff0000, // Red
0x0000ff00, // Green
0x000000ff, // Blue
};
// Loop through the colors and display them on the LED strip.
while(1)
{
for(i=0; i<3; i++)
{
memset(ledstring.channel[0].leds, 0, sizeof(ws2811_led_t) * ledstring.channel[0].count);
ledstring.channel[0].leds[0] = colors[i];
ledstring.channel[0].leds[1] = colors[i];
ledstring.channel[0].leds[2] = colors[i];
ledstring.channel[0].leds[3] = colors[i];
ledstring.channel[0].leds[4] = colors[i];
ledstring.channel[0].leds[5] = colors[i];
ledstring.channel[0].leds[6] = colors[i];
ledstring.channel[0].leds[7] = colors[i];
ws2811_render(&ledstring);
usleep(1000000);
}
}
ws2811_fini(&ledstring);
return 0;
}
```
这个程序使用了rpi_ws281x库提供的函数,通过循环控制LED灯带的颜色变化,可以通过修改colors数组中的颜色值来改变LED灯带的显示效果。
4. 编译和运行C语言程序,控制LED灯带的显示效果。可以使用gcc命令编译程序,例如:
```shell
gcc -o led_control led_control.c -lws2811
```
然后使用sudo权限运行程序,例如:
```shell
sudo ./led_control
```
这样就可以实现通过树莓派C语言控制ws2812灯带的效果了。
相关推荐
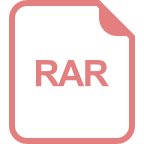














