python多条曲线呈现在一张图
时间: 2024-02-22 20:57:31 浏览: 23
你可以使用Matplotlib库中的pyplot模块来实现多条曲线在一张图中呈现。
以下是一份示例代码:
```python
import matplotlib.pyplot as plt
# 准备数据
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 2, 3, 4, 5]
y3 = [5, 4, 3, 2, 1]
# 绘制图像
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
plt.plot(x, y3, label='Line 3')
# 添加标签和标题
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Multiple Lines in One Figure')
# 添加图例
plt.legend()
# 显示图像
plt.show()
```
在这个示例中,我们定义了三条曲线的数据(y1、y2和y3),并通过plt.plot()方法在同一张图上绘制了它们。我们还添加了标签、标题和图例,最后使用plt.show()方法显示图像。
你可以根据自己的需求修改代码和数据,以便绘制出符合你要求的图像。
相关问题
python一张图画多条曲线
在Python中,可以使用Matplotlib库来绘制多条曲线。以下是一个简单的例子:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
# 绘制图像
plt.plot(x, y1, label='sin(x)')
plt.plot(x, y2, label='cos(x)')
plt.plot(x, y3, label='tan(x)')
# 添加图例
plt.legend()
# 显示图像
plt.show()
```
在这个例子中,我们生成了三组数据 `y1`、`y2`、`y3`,然后使用 `plt.plot()` 函数分别绘制了三条曲线。`label` 参数用于指定每条曲线的标签,然后使用 `plt.legend()` 函数添加图例。最后使用 `plt.show()` 函数显示图像。
你可以根据需要修改数据和标签,绘制出你需要的多条曲线。
python多条曲线
可以使用Matplotlib库来实现Python中的多条曲线绘制。具体实现方法如下所示:
```python
import matplotlib.pyplot as plt
import numpy as np
# 设置横轴的取值点
x = np.linspace(-3, 3, 50)
# 曲线1
y1 = 2 * x + 1
# 曲线2
y2 = x ** 2
# 绘制图像
plt.figure(num=3, figsize=(8, 5))
plt.plot(x, y2)
plt.plot(x, y1, color='red', linewidth=1, linestyle='--')
plt.show()
```
以上代码中,我们使用`numpy`库生成了横轴的取值点,然后分别定义了两条曲线的函数表达式,最后使用`matplotlib`库的`plot()`函数绘制出了这两条曲线。
相关推荐
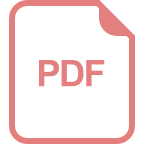












