安卓 体温监测计的数据库代码和蓝牙代码组合
时间: 2023-07-02 07:21:37 浏览: 86
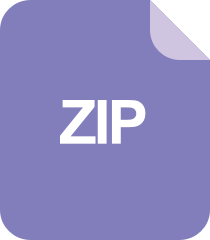
基于Android和蓝牙的单片机温度采集系统源码

以下是一个简单的安卓体温监测计应用的数据库和蓝牙代码组合示例:
```
// 数据库操作类
public class TemperatureDatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "temperature.db";
private static final int DATABASE_VERSION = 1;
private static final String TABLE_TEMPERATURE = "temperature";
private static final String COLUMN_ID = "_id";
private static final String COLUMN_TEMPERATURE_VALUE = "temperature_value";
private static final String COLUMN_TEMPERATURE_TIME = "temperature_time";
private static final String CREATE_TABLE_TEMPERATURE = "CREATE TABLE " + TABLE_TEMPERATURE + "("
+ COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT,"
+ COLUMN_TEMPERATURE_VALUE + " REAL NOT NULL,"
+ COLUMN_TEMPERATURE_TIME + " INTEGER NOT NULL);";
public TemperatureDatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_TABLE_TEMPERATURE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_TEMPERATURE);
onCreate(db);
}
}
// 蓝牙连接类
public class BluetoothConnection {
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice device;
private BluetoothSocket socket;
private InputStream inputStream;
private OutputStream outputStream;
public BluetoothConnection(String deviceAddress) {
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
device = bluetoothAdapter.getRemoteDevice(deviceAddress);
}
public boolean connect() {
try {
socket = device.createRfcommSocketToServiceRecord(UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"));
socket.connect();
inputStream = socket.getInputStream();
outputStream = socket.getOutputStream();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public void disconnect() {
try {
socket.close();
inputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public float readData() {
byte[] buffer = new byte[1024];
int bytes;
try {
bytes = inputStream.read(buffer);
String data = new String(buffer, 0, bytes);
return Float.parseFloat(data);
} catch (IOException e) {
e.printStackTrace();
return -1f;
}
}
public void writeData(float data) {
try {
outputStream.write(String.valueOf(data).getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
}
// 主界面代码
public class MainActivity extends AppCompatActivity {
private TextView temperatureTextView;
private TemperatureDatabaseHelper databaseHelper;
private BluetoothConnection bluetoothConnection;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
temperatureTextView = findViewById(R.id.temperature_text_view);
databaseHelper = new TemperatureDatabaseHelper(this);
bluetoothConnection = new BluetoothConnection("00:11:22:33:AA:BB");
if (bluetoothConnection.connect()) {
Toast.makeText(this, "Bluetooth connected", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(this, "Bluetooth connection failed", Toast.LENGTH_SHORT).show();
}
new Thread(new Runnable() {
@Override
public void run() {
while (true) {
float temperature = bluetoothConnection.readData();
if (temperature >= 0f) {
databaseHelper.getWritableDatabase().execSQL("INSERT INTO temperature(temperature_value, temperature_time) VALUES(" + temperature + ", " + System.currentTimeMillis() + ");");
runOnUiThread(new Runnable() {
@Override
public void run() {
temperatureTextView.setText(String.format(Locale.getDefault(), "%.1f", temperature));
}
});
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
@Override
protected void onDestroy() {
super.onDestroy();
bluetoothConnection.disconnect();
}
}
```
这个示例中,我们使用了一个基于 SQLite 的本地数据库来存储温度数据,通过蓝牙与设备连接并读取数据,将数据存储到数据库中,并将最新的温度显示在界面上。需要注意的是,这只是一个简单示例,实际应用中需要更加完善和健壮的错误处理和数据管理机制。
阅读全文
相关推荐
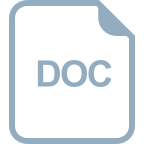
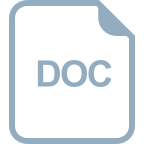

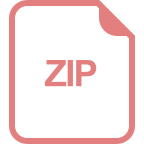
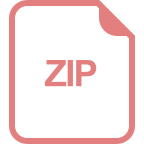
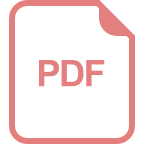
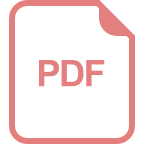
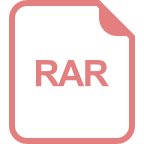
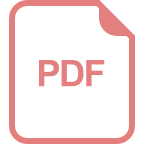
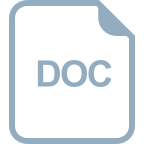
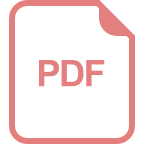
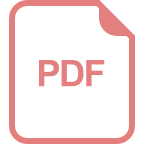
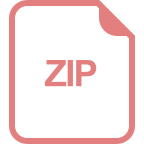
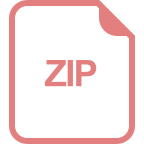
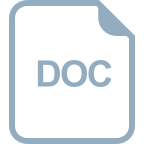