c#遍历整个hashtable
时间: 2024-10-26 12:01:50 浏览: 24
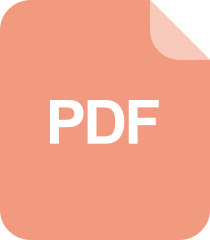
C#中遍历Hashtable的4种方法
在C#中,你可以使用`foreach`循环来遍历`Hashtable`(在.NET Framework中已被`Dictionary<TKey, TValue>`取代),这是一种关联数组数据结构。以下是遍历`Dictionary`的示例:
```csharp
Dictionary<string, int> myHashTable = new Dictionary<string, int>
{
{ "Key1", 1 },
{ "Key2", 2 },
{ "Key3", 3 }
};
foreach (KeyValuePair<string, int> pair in myHashTable)
{
string key = pair.Key; // 当前键
int value = pair.Value; // 对应的值
Console.WriteLine($"Key: {key}, Value: {value}");
}
```
在这个例子中,`KeyValuePair<TKey, TValue>`是一个泛型结构,代表字典中的键值对。每次迭代,`pair.Key`将获取当前键,`pair.Value`获取对应的值。
阅读全文
相关推荐
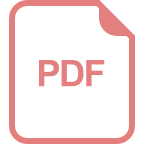
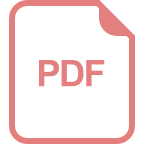


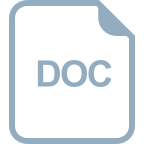

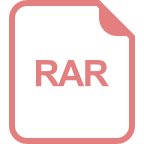
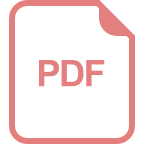
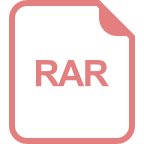
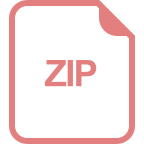
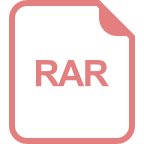
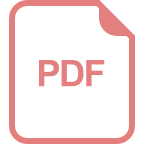



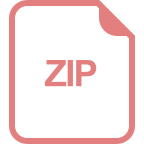