c++先序创建二叉树。按先序次序输入,以#字符表示空树
时间: 2024-11-18 15:19:22 浏览: 13
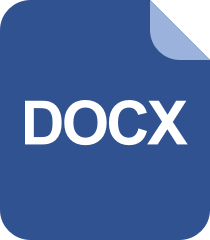
数据结构实验报告8-树-求二叉树先序、中序、后序遍历次序的第k个访问结点-实验内容与要求.docx
在C++中,我们可以使用递归的方式来构建先序遍历输入的二叉树。先序遍历的顺序通常是根节点 -> 左子树 -> 右子树。如果你收到一个以'#'表示空节点的字符串,可以按照以下步骤转换成二叉树结构:
1. 定义一个Node类,包含一个值和两个指向左右孩子的指针(默认为空)。
```cpp
class Node {
public:
char value;
Node* left = nullptr;
Node* right = nullptr;
};
```
2. 创建一个函数`buildTree(string inorder, string postorder)`, 先序和后序遍历作为参数。这两个字符串分别代表了元素在先序遍历和后序遍历中的相对位置。
3. 使用后序遍历的最后一个元素作为当前根节点,找到它在先序遍历中的位置,然后从先序遍历中删除该节点,将剩余部分继续分割为左子树和右子树。
```cpp
Node* buildTree(string inorder, string postorder) {
if (inorder.empty()) return nullptr;
// 获取后序遍历中的根节点
int index = postorder.find('#');
if (index == -1) {
// 如果后序遍历中没有#, 说明这是一个叶子节点
Node* root = new Node{postorder.back()};
postorder.pop_back();
inorder.pop_back(); // 删除先序遍历中的最后一个元素
return root;
}
// 根据先序遍历找到根节点
char rootValue = inorder[index];
Node* root = new Node{rootValue};
// 分别构建左子树和右子树
int i = 0;
for (; i < inorder.size() && inorder[i] != rootValue; ++i)
; // 找到根节点的位置
// 递归构建左子树和右子树
root->left = buildTree(inorder.substr(0, i), postorder.substr(0, index));
root->right = buildTree(inorder.substr(i + 1), postorder.substr(index + 1));
return root;
}
```
4. 调用`buildTree()`函数,传入先序遍历和后序遍历的字符串作为参数,得到二叉树的根节点。
例如,给定先序遍历序列 "A-B-D-E-C-F" 和后序遍历序列 "DBEFAC",可以构建出如下的二叉树:
```
A
/ \
B C
/ \ / \
D E F #
```
阅读全文
相关推荐
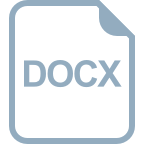
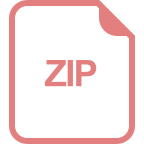

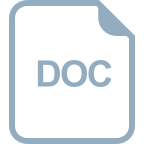
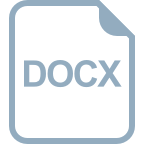
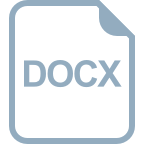
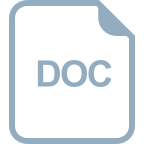
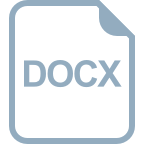
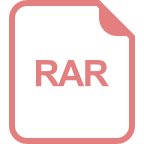
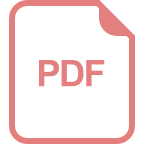
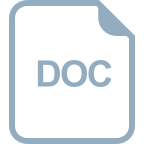
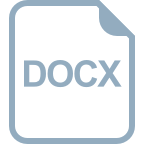
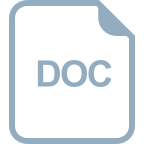
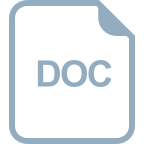




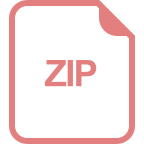