帮我用c语言实现这个程序,程序功能:射击比赛。输入6个射击比赛选手的编号(整数)和成绩,找出最高和最低射击成绩的比赛选手并输出,成绩相同时,输出最先输入的选手编号。 输入格式: 依次输入选手编号和成绩。 输出格式: 输出最高和最低的射击成绩及其选手编号。
时间: 2024-10-28 21:06:12 浏览: 7
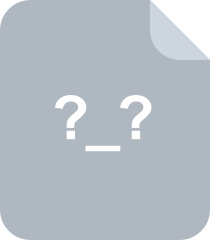
c语言编写程序实现两个超长正整数(每个最长80位数字)的减法运算
在C语言中,你可以使用数组和结构体来实现这个射击比赛程序。首先,我们需要定义一个结构体表示选手信息,包括选手编号和成绩,然后定义两个全局变量分别存储最高分和最低分以及对应的选手编号。以下是详细的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义选手信息结构体
typedef struct {
int id;
int score;
} Shooter;
// 初始化最高分和最低分
Shooter highest = {0, 0};
Shooter lowest = {0, 9999};
void inputScores() {
int n, score;
printf("请输入选手编号和成绩(按顺序输入,格式如:1 80),输入结束请输入0\n");
while (scanf("%d %d", &n, &score) == 2 && n != 0) {
Shooter shooter = {n, score};
// 更新最高分和最低分
if (score > highest.score || (score == highest.score && n < highest.id)) {
highest = shooter;
} else if (score < lowest.score || (score == lowest.score && n > lowest.id)) {
lowest = shooter;
}
}
}
void printResults() {
printf("最高得分: %d 分,选手编号: %d\n", highest.score, highest.id);
printf("最低得分: %d 分,选手编号: %d\n", lowest.score, lowest.id);
}
int main() {
inputScores();
printResults();
return 0;
}
```
在这个程序中,我们先定义了一个`inputScores`函数用于读取用户输入,并更新最高分和最低分。`printResults`函数则用于打印结果。记得运行前,确保已经设置了正确的输入格式。
阅读全文
相关推荐
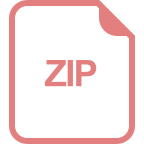
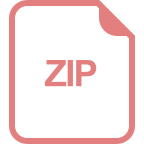
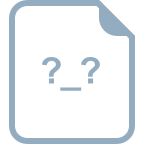
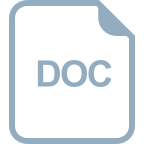
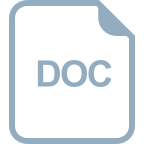
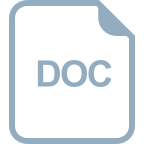
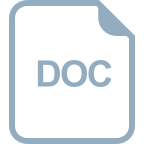
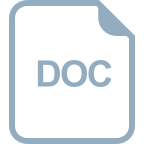
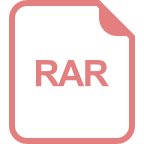
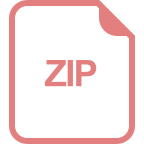
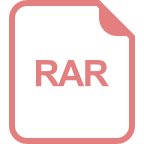
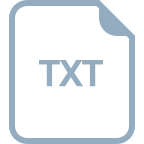
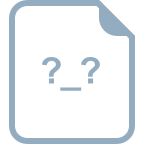
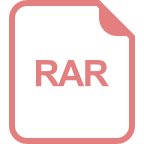