C++:从键盘输入几名学生的姓名和年龄,输入时,在单独的一行中按Ctrl+Z组合键再按回车键以结束输入。(假设学生姓名中都没有空格)以二进制文件形式存储该程序,生成一个学生记录文件student.dat
时间: 2024-12-04 20:23:50 浏览: 30
在C++中,你可以使用标准库中的`fstream`来创建二进制文件并保存学生信息。这里是一个简单的示例,展示了如何从键盘输入学生的信息并以二进制格式保存至文件`student.dat`:
```cpp
#include <fstream>
#include <string>
#include <vector>
#include <limits> // 获取std::numeric_limits<int>::max()用于获取最大整数值
std::vector<std::pair<std::string, int>> students; // 存储学生信息
void inputStudents() {
while (true) {
std::string name;
int age;
std::cout << "Enter student's name or Ctrl+Z followed by Enter to finish: ";
std::getline(std::cin, name);
if (name == "<Ctrl-Z>") break; // 判断是否按下Ctrl+Z
std::cin >> age; // 输入年龄,注意这将跳过回车符
if (age > std::numeric_limits<int>::max()) {
std::cerr << "Age exceeds the maximum limit. Skipping this entry.\n";
continue;
}
students.push_back({name, age}); // 存储学生信息
}
}
void saveToBinaryFile() {
std::ofstream output("student.dat", std::ios::binary);
if (!output) {
std::cerr << "Failed to open the output file for writing.\n";
return;
}
for (const auto& [name, age] : students) {
output.write(name.c_str(), name.length()); // 写名字
output.write(reinterpret_cast<char*>(&age), sizeof(int)); // 写年龄
}
output.close();
std::cout << "Student data saved in binary format to student.dat.\n";
}
int main() {
inputStudents();
saveToBinaryFile();
return 0;
}
```
当用户输入完所有学生的信息后,程序会关闭文件并提示“Student data saved in binary format to student.dat”。
阅读全文
相关推荐
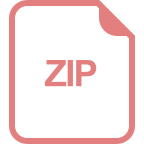
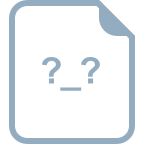
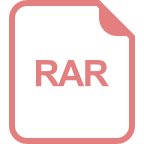
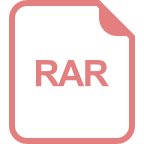
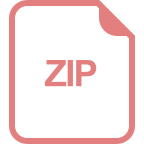
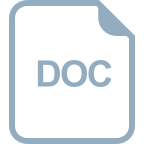
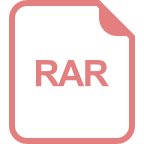
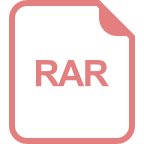
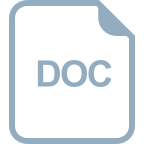
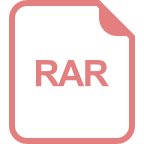
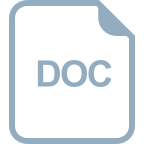
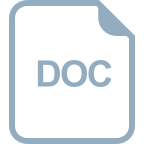
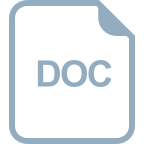
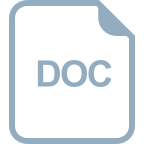
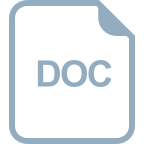
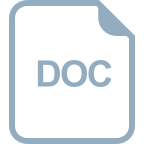
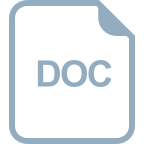
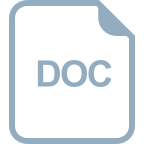