inference.py在models文件夹中,utils.py在dataset文件夹中,两个文件夹同在SAM文件夹中,inference.py想引用utils.py的generate_test_loader和generate_dataset两个函数,该怎么引用
时间: 2024-04-11 16:28:37 浏览: 128
对于inference.py想要引用utils.py中的函数,可以使用相对导入。假设你的文件结构如下所示:
```
SAM/
├── models/
│ └── inference.py
└── dataset/
└── utils.py
```
在inference.py中,你可以使用以下方式引用utils.py中的函数:
```python
from ..dataset.utils import generate_test_loader, generate_dataset
```
这样就可以成功导入utils.py中的函数了。需要注意的是,使用相对导入时,需要确保两个文件夹处于同一级别下,否则需要相应地调整导入路径。
相关问题
yolov5 detect.py中打开摄像头的代码在哪
在YoloV5的`detect.py`中,打开摄像头的代码可以在`detect()`函数中找到,具体代码如下:
```python
def detect():
...
# 如果使用摄像头,打开摄像头并获取视频流
if opt.source == '0' or opt.source.startswith('rtsp') or opt.source.startswith('http'):
# 打开摄像头或者视频流
view_img = True
cudnn.benchmark = True # set True to speed up constant image size inference
dataset = LoadStreams(opt.source, img_size=imgsz)
else:
# 读取本地视频文件
save_img = True
dataset = LoadImages(opt.source, img_size=imgsz)
# 读取模型
model = attempt_load(weights, map_location=device) # load FP32 model
imgsz = check_img_size(imgsz, s=model.stride.max()) # check img_size
if half:
model.half() # to FP16
# 获取类别名称
names = model.module.names if hasattr(model, 'module') else model.names
# 进行推理
results = []
for path, img, im0s, vid_cap in dataset:
# img : 当前帧的缩放后的图片
# im0s : 当前帧的原图
# 进行检测
t1 = torch_utils.time_synchronized()
img = torch.from_numpy(img).to(device)
img = img.half() if half else img.float()
img /= 255.0 # 0 - 255 to 0.0 - 1.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 获取预测结果
pred = model(img, augment=opt.augment)[0]
# 进行后处理
pred = non_max_suppression(pred, conf_thres=conf_thres, iou_thres=iou_thres, classes=opt.classes,
agnostic=agnostic_nms, max_det=max_det)
t2 = torch_utils.time_synchronized()
# 输出当前帧信息
for i, det in enumerate(pred): # detections per image
if webcam: # batch_size >= 1
p, s, im0 = path[i], '%g: ' % i, im0s[i]
else:
p, s, im0 = path, '', im0s
save_path = str(Path(out) / Path(p).name)
txt_path = str(Path(out) / Path(p).stem) + (f'_{frame_i:06d}' if save_img else '')
s += '%gx%g ' % img.shape[2:] # print string
gn = torch.tensor(im0.shape)[[1, 0, 1, 0]] # normalization gain whwh
if det is not None and len(det):
# Rescale boxes from img_size to im0 size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0.shape).round()
# Print results
for c in det[:, -1].unique():
n = (det[:, -1] == c).sum() # detections per class
s += f"{n} {names[int(c)]}{'s' * (n > 1)}, " # add to string
# Write results
for *xyxy, conf, cls in det:
if save_txt: # Write to file
xywh = (xyxy2xywh(torch.tensor(xyxy).view(1, 4)) / gn).view(-1).tolist() # normalized xywh
with open(txt_path + '.txt', 'a') as f:
f.write(('%g ' * 5 + '\n') % (cls, *xywh)) # label format
if save_img or view_img: # Add bbox to image
label = f'{names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, im0, label=label, color=colors[int(cls)], line_thickness=3)
# Print time (inference + NMS)
print(f'{s}Done. ({t2 - t1:.3f}s)')
# Stream results
if view_img:
cv2.imshow(str(p), im0)
if cv2.waitKey(1) == ord('q'): # q to quit
raise StopIteration
# Save results (image with detections)
if save_img:
if dataset.mode == 'images':
cv2.imwrite(save_path, im0)
print(f'Done. ({time.time() - t0:.3f}s)')
```
在上面的代码中,如果`opt.source`为`0`或者以`rtsp`或`http`开头,则表示打开摄像头或视频流,代码中会调用`LoadStreams`函数加载视频流。在`for path, img, im0s, vid_cap in dataset:`这一行代码中,`img`表示当前帧的缩放后的图片,`im0s`表示当前帧的原图。在代码中,会对当前帧的图片进行目标检测,并对检测结果进行后处理,最后将结果输出到屏幕上或保存到本地。如果需要显示视频流,则调用`cv2.imshow`函数将当前帧的原图显示到屏幕上。
yolov5中detect.py的核心代码是什么
YOLOv5(You Only Look Once version 5)是一个流行的实时物体检测模型,其`detect.py`文件核心部分主要包括模型加载、图像处理、前向传播以及结果解析。以下是该脚本中的关键代码段:
```python
# 导入必要的模块
from models.experimental import attempt_load
import torch
from utils.datasets import LoadImages
from utils.general import check_img_size, non_max_suppression
# 加载预训练模型
model = attempt_load('yolov5s.pt', map_location='cpu') # 指定模型路径和设备(CPU)
# 定义输入图像的处理函数
def inference(imgsz=640, conf_thres=0.25, iou_thres=0.45):
device = next(model.parameters()).device # 获取设备
dataset = LoadImages('image.jpg', img_size=imgsz) # 创建数据集实例
for path, img, im0s, vid_cap in dataset: # 遍历图片
img = torch.from_numpy(img).to(device) # 将图像转为Tensor并移动到设备上
pred = model(img)[0] # 前向推理得到预测结果
pred = non_max_suppression(pred, conf_thres, iou_thres) # NMS处理
result = [[bbox.tolist(), cls_id.item()] for bbox, cls_id in pred] # 可视化所需的框坐标和类别
...
# 调用inference函数进行实际检测
inference()
```
这部分代码首先加载预训练模型,然后设置好输入图像的大小(imgsz),confidence阈值(conf_thres)和IoU阈值(iou_thres)。接着,它读取单张图像或视频流,并对每帧进行预测,通过非极大值抑制(NMS)筛选出最终的检测结果。
阅读全文
相关推荐
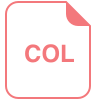
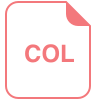
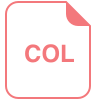
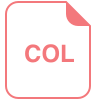
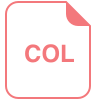
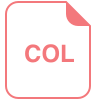
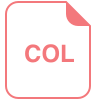
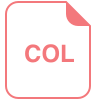
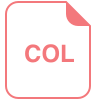
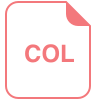



