最优装载问题用贪心算法求解
时间: 2024-04-14 08:24:26 浏览: 14
最优装载问题是一个经典的组合优化问题,它的目标是在给定一组物品和一个容量限制的背包中,找到一种装载方式使得背包中的物品总重量最大化。
贪心算法是一种常用的求解最优装载问题的方法。它的基本思想是每次选择当前看起来最优的物品放入背包中,直到背包无法再装下任何物品为止。
具体的贪心算法求解最优装载问题的步骤如下:
1. 将物品按照单位重量的价值从大到小进行排序。
2. 依次将排序后的物品放入背包中,直到背包无法再装下任何物品或者所有物品都已经放入背包为止。
以下是一个用贪心算法求解最优装载问题的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct Item {
int weight;
int value;
};
bool compare(Item a, Item b) {
return (a.value / a.weight) > (b.value / b.weight);
}
double knapsack(int capacity, std::vector<Item>& items) {
std::sort(items.begin(), items.end(), compare);
double totalValue = 0.0;
int currentWeight = 0;
for (int i = 0; i < items.size(); i++) {
if (currentWeight + items[i].weight <= capacity) {
currentWeight += items[i].weight;
totalValue += items[i].value;
} else {
int remainingCapacity = capacity - currentWeight;
totalValue += items[i].value * (static_cast<double>(remainingCapacity) / items[i].weight);
break;
}
}
return totalValue;
}
int main() {
int capacity = 50;
std::vector<Item> items = {{10, 60}, {20, 100}, {30, 120}};
double maxValue = knapsack(capacity, items);
std::cout << "The maximum value that can be loaded into the knapsack is: " << maxValue << std::endl;
return 0;
}
```
在上述代码中,我们定义了一个`Item`结构体来表示物品的重量和价值。然后,我们实现了一个比较函数`compare`,用于将物品按照单位重量的价值从大到小进行排序。接下来,我们实现了`knapsack`函数来求解最优装载问题。最后,在`main`函数中,我们定义了一个背包容量和一组物品,并调用`knapsack`函数来求解最大装载价值。
需要注意的是,贪心算法并不一定能够得到最优解,它只能得到一个近似的解。在某些情况下,贪心算法可能会得到次优解甚至是错误的解。因此,在实际应用中,需要根据具体问题的特点来选择合适的算法。
相关推荐
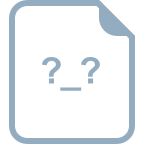
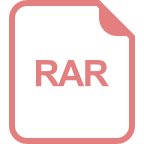
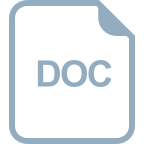














