springboot mybatis-plus多表关联查询
时间: 2023-09-18 18:09:03 浏览: 131
好的,关于这个问题,我可以告诉你,在Spring Boot中,可以使用MyBatis-Plus进行多表关联查询。使用MyBatis-Plus的Wrapper类,可以很方便地实现多表关联查询。例如,可以使用Join方法连接表,或者使用LambdaQueryWrapper和LambdaUpdateWrapper等方法进行复杂的多表查询和更新操作。另外,MyBatis-Plus还提供了一些方便的注解,比如@TableJoin注解和@ColumnJoin注解,可以更便捷地定义多表关联查询语句。希望这些信息对你有所帮助。
相关问题
springboot+mybatis-plus联合查询
Spring Boot 是一个快速开发框架,Mybatis-Plus 是 Mybatis 的增强工具,它们两个结合使用可以让我们更加方便的进行数据持久化。联合查询指的是通过关联多张表的方式,一次性查询出多张表中的数据,通常可以使用 Mybatis-Plus 提供的 Wrapper 来实现联合查询,以下是 Spring Boot + Mybatis-Plus 联合查询的一个例子:
假设有两张表:user 和 order,user 表中有一个 user_id 字段,order 表中有一个 user_id 字段。现在需要查询某个用户的所有订单信息,可以使用以下代码:
```
public List<Order> getUserOrders(Long userId) {
QueryWrapper<User> userQueryWrapper = new QueryWrapper<>();
userQueryWrapper.eq("user_id", userId);
User user = userMapper.selectOne(userQueryWrapper);
QueryWrapper<Order> orderQueryWrapper = new QueryWrapper<>();
orderQueryWrapper.eq("user_id", userId);
List<Order> orders = orderMapper.selectList(orderQueryWrapper);
for (Order order : orders) {
order.setUser(user);
}
return orders;
}
```
这里使用了 Mybatis-Plus 提供的 QueryWrapper 对象来构建查询条件,然后分别调用 userMapper 和 orderMapper 的 selectOne 和 selectList 方法来查询数据,最后将查询到的 User 对象设置到每个 Order 对象中。
springboot+mybatis-plus实现两个数据库的两张表相连查询数据
可以使用Mybatis-Plus提供的多数据源配置,然后在查询时指定使用哪个数据源。以下是示例代码:
1. 配置多数据源
在application.yml文件中配置两个数据源:
```yaml
spring:
datasource:
master:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/db1?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC
username: root
password: root
slave:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/db2?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC
username: root
password: root
```
在配置类中添加多数据源配置:
```java
@Configuration
@MapperScan(basePackages = "com.example.mapper")
public class DataSourceConfig {
@Bean("master")
@ConfigurationProperties("spring.datasource.master")
public DataSource masterDataSource() {
return DataSourceBuilder.create().build();
}
@Bean("slave")
@ConfigurationProperties("spring.datasource.slave")
public DataSource slaveDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
public SqlSessionFactory sqlSessionFactory(@Qualifier("master") DataSource masterDataSource,
@Qualifier("slave") DataSource slaveDataSource) throws Exception {
SqlSessionFactoryBean sessionFactory = new SqlSessionFactoryBean();
sessionFactory.setDataSource(routingDataSource(masterDataSource, slaveDataSource));
ResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
sessionFactory.setMapperLocations(resolver.getResources("classpath*:mapper/**/*.xml"));
return sessionFactory.getObject();
}
@Bean
public DataSource routingDataSource(@Qualifier("master") DataSource masterDataSource,
@Qualifier("slave") DataSource slaveDataSource) {
Map<Object, Object> dataSourceMap = new HashMap<>();
dataSourceMap.put("master", masterDataSource);
dataSourceMap.put("slave", slaveDataSource);
RoutingDataSource routingDataSource = new RoutingDataSource();
routingDataSource.setDefaultTargetDataSource(masterDataSource);
routingDataSource.setTargetDataSources(dataSourceMap);
return routingDataSource;
}
}
```
2. 实现两个表的关联查询
在mapper中编写SQL语句,可以使用Mybatis-Plus提供的Wrapper类来实现多条件查询。
```java
@Mapper
public interface UserMapper extends BaseMapper<User> {
@Select("SELECT * FROM user u JOIN order o ON u.id = o.user_id WHERE u.id = #{userId}")
@Results({
@Result(column = "id", property = "id"),
@Result(column = "username", property = "username"),
@Result(column = "id", property = "orders", many = @Many(select = "com.example.mapper.OrderMapper.selectByUserId")),
})
User selectUserWithOrders(@Param("userId") Long userId);
}
@Mapper
public interface OrderMapper extends BaseMapper<Order> {
@Select("SELECT * FROM order WHERE user_id = #{userId}")
List<Order> selectByUserId(Long userId);
}
```
在service中调用mapper方法即可实现两个表的关联查询。
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserWithOrders(Long userId) {
return userMapper.selectUserWithOrders(userId);
}
}
```
阅读全文
相关推荐
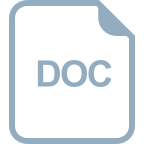
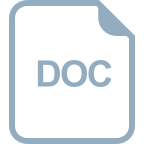
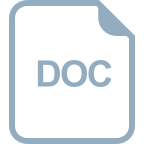
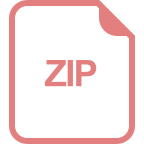
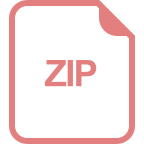
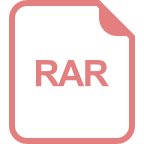
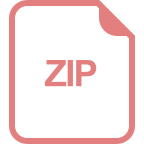
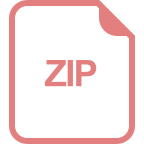
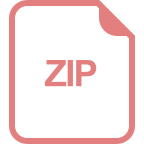
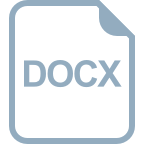
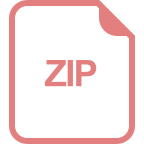
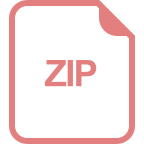
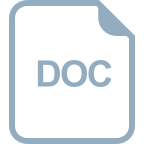
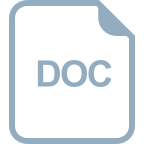
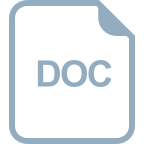

