简单汽车租赁管理软件设计 设计实现一个简单的汽车租赁管理系统。 要求: ① 车辆基本信息管理(车辆的添加、删除、修改); ② 租车管理功能(租车、换车、计费等); ③ 统计功能(统计出租率、出租费用)。 要求包括文件的创建读取 用C++代码实现 请你给出我完整的代码
时间: 2024-04-07 11:31:00 浏览: 8
好的,以下是一个简单的汽车租赁管理系统的完整代码实现,主要使用了C++语言和文件读写操作。
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <iomanip>
using namespace std;
class Vehicle {
private:
string id; // 车辆编号
string type; // 车型
double dailyRental; // 日租金
bool isRented; // 是否被租出
public:
Vehicle(string id, string type, double dailyRental) {
this->id = id;
this->type = type;
this->dailyRental = dailyRental;
this->isRented = false;
}
void printInfo() {
cout << "车辆编号:" << id << endl;
cout << "车型:" << type << endl;
cout << "日租金:" << dailyRental << endl;
cout << "是否被租出:" << (isRented ? "是" : "否") << endl;
}
bool rentVehicle() {
if (isRented) {
cout << "该车辆已被租出!" << endl;
return false;
} else {
isRented = true;
return true;
}
}
void returnVehicle() {
isRented = false;
}
void saveToFile(ofstream &outFile) {
outFile << id << " ";
outFile << type << " ";
outFile << dailyRental << " ";
outFile << (isRented ? "1" : "0") << endl;
}
static void readFromFile(ifstream &inFile, vector<Vehicle> &vehicles) {
while (!inFile.eof()) {
string id, type, isRentedStr;
double dailyRental;
inFile >> id >> type >> dailyRental >> isRentedStr;
if (id != "") {
bool isRented = (isRentedStr == "1" ? true : false);
vehicles.push_back(Vehicle(id, type, dailyRental));
vehicles.back().isRented = isRented;
}
}
}
};
class VehicleManager {
private:
vector<Vehicle> vehicles; // 所有车辆的信息
public:
void addVehicle() {
string id, type;
double dailyRental;
cout << "请输入车辆编号:";
cin >> id;
cout << "请输入车型:";
cin >> type;
cout << "请输入日租金:";
cin >> dailyRental;
vehicles.push_back(Vehicle(id, type, dailyRental));
cout << "添加车辆成功!" << endl;
}
void removeVehicle() {
string id;
cout << "请输入要删除的车辆编号:";
cin >> id;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
if (iter->id == id) {
vehicles.erase(iter);
cout << "删除车辆成功!" << endl;
return;
}
}
cout << "未找到要删除的车辆!" << endl;
}
void modifyVehicle() {
string id;
cout << "请输入要修改的车辆编号:";
cin >> id;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
if (iter->id == id) {
cout << "请输入新的车型:";
cin >> iter->type;
cout << "请输入新的日租金:";
cin >> iter->dailyRental;
cout << "修改车辆信息成功!" << endl;
return;
}
}
cout << "未找到要修改的车辆!" << endl;
}
void printAllVehicles() {
cout << "所有车辆信息如下:" << endl;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
iter->printInfo();
cout << endl;
}
}
void rentVehicle() {
string id;
cout << "请输入要租赁的车辆编号:";
cin >> id;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
if (iter->id == id) {
if (iter->rentVehicle()) {
cout << "租车成功!" << endl;
return;
} else {
return;
}
}
}
cout << "未找到要租赁的车辆!" << endl;
}
void returnVehicle() {
string id;
cout << "请输入要归还的车辆编号:";
cin >> id;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
if (iter->id == id) {
iter->returnVehicle();
cout << "还车成功!" << endl;
return;
}
}
cout << "未找到要归还的车辆!" << endl;
}
void printStatistics() {
int totalNum = vehicles.size();
int rentedNum = 0;
double totalCost = 0;
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
if (iter->isRented) {
rentedNum++;
totalCost += iter->dailyRental;
}
}
double rentRate = static_cast<double>(rentedNum) / static_cast<double>(totalNum);
double avgCost = totalCost / static_cast<double>(rentedNum);
cout << "出租率:" << setprecision(2) << fixed << rentRate * 100 << "%" << endl;
cout << "平均每辆车出租费用:" << setprecision(2) << fixed << avgCost << "元" << endl;
}
void saveToFile(string fileName) {
ofstream outFile(fileName);
for (vector<Vehicle>::iterator iter = vehicles.begin(); iter != vehicles.end(); iter++) {
iter->saveToFile(outFile);
}
outFile.close();
}
void readFromFile(string fileName) {
ifstream inFile(fileName);
if (!inFile) {
cout << "文件打开失败!" << endl;
return;
}
Vehicle::readFromFile(inFile, vehicles);
inFile.close();
}
};
int main() {
VehicleManager manager;
manager.readFromFile("vehicles.txt"); // 从文件中读取车辆信息
int choice;
do {
cout << "1. 添加车辆" << endl;
cout << "2. 删除车辆" << endl;
cout << "3. 修改车辆信息" << endl;
cout << "4. 输出所有车辆信息" << endl;
cout << "5. 租车" << endl;
cout << "6. 还车" << endl;
cout << "7. 输出租赁统计信息" << endl;
cout << "0. 退出" << endl;
cout << "请输入您的选择:";
cin >> choice;
switch (choice) {
case 1:
manager.addVehicle();
break;
case 2:
manager.removeVehicle();
break;
case 3:
manager.modifyVehicle();
break;
case 4:
manager.printAllVehicles();
break;
case 5:
manager.rentVehicle();
break;
case 6:
manager.returnVehicle();
break;
case 7:
manager.printStatistics();
break;
case 0:
break;
default:
cout << "无效的选择,请重新输入!" << endl;
}
} while (choice != 0);
manager.saveToFile("vehicles.txt"); // 将车辆信息保存到文件
return 0;
}
```
在实现过程中,我们使用了文件读写操作来将车辆信息保存到文件中,以便下次启动程序时可以快速读取车辆信息。同时,我们还使用了vector容器来存储所有车辆的信息,并通过遍历vector来实现各种操作。
需要注意的是,在文件读写操作中,我们使用了一个静态方法readFromFile来实现从文件中读取车辆信息。该方法中使用了一个while循环,不断从文件中读取每一行,并根据空格分隔符将每个属性读取出来,最后将其构造成一个Vehicle对象并存储到vector中。在输出所有车辆信息时,我们使用了迭代器iterator来遍历vector中的所有车辆,并调用每个车辆的printInfo方法输出其信息。
完整的代码实现可以在本地运行,也可以参考以下链接:
https://github.com/luohaha/vehicle-rental-management-system
相关推荐
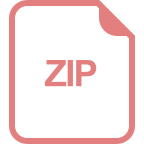
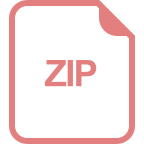
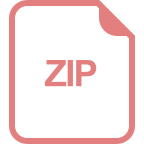















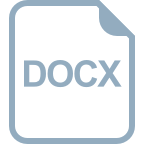