jsp+servlet实现获取当前天气
时间: 2023-11-05 10:05:08 浏览: 161
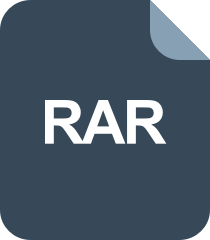
jsp实现实时天气预报

要通过JSP和Servlet获取当前天气,需要使用一些API来获取天气信息。以下是实现的一般步骤:
1. 注册并获取天气API的访问密钥,例如使用百度API Store提供的免费天气API。
2. 在JSP页面中引入jQuery库和自定义的JavaScript脚本文件,用于实现异步获取天气信息并更新页面。
3. 在JSP页面中添加一个用于显示天气信息的HTML元素,例如标签。
4. 在自定义的JavaScript脚本文件中编写异步请求天气API的方法,并处理返回的JSON格式的数据。
5. 在Servlet中处理异步请求,获取天气信息并返回JSON格式的数据。
以下是一个简单的示例代码:
JSP页面代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>获取当前天气</title>
<script src="https://cdn.bootcss.com/jquery/3.2.1/jquery.min.js"></script>
<script src="getWeather.js"></script>
</head>
<body>
<h1>当前天气</h1>
<div id="weather"></div>
</body>
</html>
```
自定义JavaScript脚本文件代码(getWeather.js):
```javascript
$(document).ready(function() {
getWeather();
});
function getWeather() {
$.ajax({
url: "weather",
success: function(data) {
var weather = data.weather[0].description;
var temp = data.main.temp;
var humidity = data.main.humidity;
$("#weather").html("天气:" + weather + ",温度:" + temp + ",湿度:" + humidity);
},
error: function() {
$("#weather").html("无法获取天气信息");
}
});
}
```
Servlet代码:
```java
import java.io.IOException;
import java.io.PrintWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.json.JSONObject;
public class WeatherServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("application/json;charset=UTF-8");
PrintWriter out = response.getWriter();
String key = "your_api_key"; // 替换为你的API访问密钥
String city = "Beijing"; // 替换为你要获取天气的城市
String apiUrl = "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=" + key;
URL url = new URL(apiUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
Scanner scanner = new Scanner(conn.getInputStream());
String result = "";
while (scanner.hasNext()) {
result += scanner.nextLine();
}
scanner.close();
JSONObject json = new JSONObject(result);
out.print(json);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
```
需要注意的是,以上示例代码仅供参考,实际使用时需要根据具体情况进行适当的修改和调整。同时,也需要考虑API的访问限制和使用限制,避免超出免费使用额度或违反相关规定。
阅读全文
相关推荐
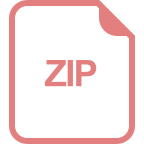
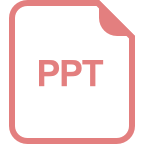
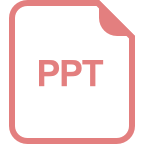
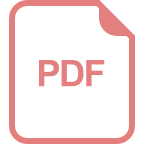
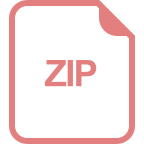
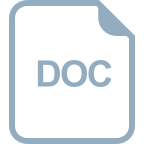
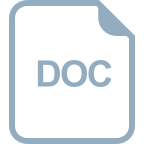
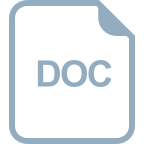

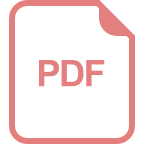
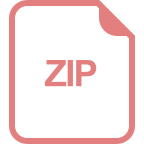
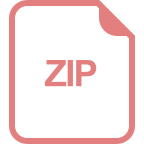
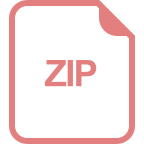
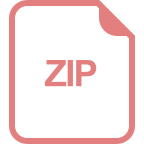
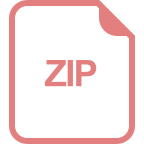