请用python写一个大鱼吃小鱼的游戏
时间: 2023-07-07 13:33:37 浏览: 388
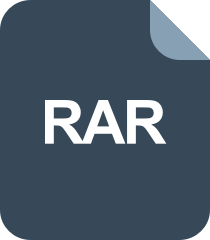
Pygame 大鱼吃小鱼

好的,以下是一个简单的大鱼吃小鱼的游戏。
```
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置窗口尺寸
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置游戏标题
pygame.display.set_caption("Big Fish Eats Small Fish")
# 加载游戏资源
fish_image = pygame.image.load("fish.png").convert_alpha()
fish_image = pygame.transform.scale(fish_image, (50, 50))
small_fish_image = pygame.image.load("small_fish.png").convert_alpha()
small_fish_image = pygame.transform.scale(small_fish_image, (30, 30))
# 设置游戏参数
fish_x = SCREEN_WIDTH / 2
fish_y = SCREEN_HEIGHT / 2
fish_speed = 5
small_fish_num = 10
small_fish_list = []
# 创建小鱼
for i in range(small_fish_num):
small_fish_x = random.randint(0, SCREEN_WIDTH - 30)
small_fish_y = random.randint(0, SCREEN_HEIGHT - 30)
small_fish_list.append((small_fish_x, small_fish_y))
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 处理键盘输入
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
fish_x -= fish_speed
if keys[pygame.K_RIGHT]:
fish_x += fish_speed
if keys[pygame.K_UP]:
fish_y -= fish_speed
if keys[pygame.K_DOWN]:
fish_y += fish_speed
# 绘制游戏元素
screen.fill((255, 255, 255))
screen.blit(fish_image, (fish_x, fish_y))
for small_fish in small_fish_list:
screen.blit(small_fish_image, small_fish)
# 碰撞检测
for small_fish in small_fish_list:
if fish_x < small_fish[0] + 30 and fish_x + 50 > small_fish[0] and fish_y < small_fish[1] + 30 and fish_y + 50 > small_fish[1]:
small_fish_list.remove(small_fish)
# 更新屏幕
pygame.display.update()
# 退出游戏
pygame.quit()
```
这个游戏非常简单,只有一个大鱼和一些小鱼。玩家可以用方向键控制大鱼的移动,吃掉所有的小鱼即可胜利。当大鱼和小鱼碰撞时,小鱼会被吃掉。
阅读全文
相关推荐




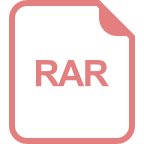
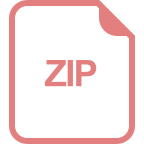









