vue 淘宝足迹功能 代码实现
时间: 2023-08-15 17:10:37 浏览: 136
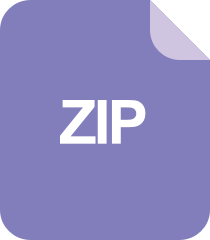
实现淘宝功能的源代码
好的,下面是一个简单的 Vue 淘宝足迹功能的代码实现,供您参考:
1. 创建足迹组件 Footprint.vue
```html
<template>
<div>
<h1>我的足迹</h1>
<div class="footprint-list">
<div v-for="item in footprintList" :key="item.id" class="footprint-item" @click="goDetail(item.id)">
<img :src="item.img" alt="">
<p>{{ item.name }}</p>
<span>{{ item.price }}</span>
</div>
</div>
<div class="add-footprint">
<input type="text" placeholder="请输入商品ID" v-model="productId">
<button @click="addFootprint">添加足迹</button>
</div>
</div>
</template>
<script>
import { mapState, mapActions } from 'vuex'
export default {
computed: {
...mapState(['footprintList'])
},
methods: {
...mapActions(['addFootprint']),
goDetail(id) {
this.$router.push(`/detail/${id}`)
}
},
data() {
return {
productId: ''
}
}
}
</script>
```
2. 在 store.js 中创建足迹列表的状态和添加足迹的 action
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
footprintList: []
},
mutations: {
addFootprint(state, item) {
state.footprintList.unshift(item)
}
},
actions: {
async addFootprint({ commit }, productId) {
// 调用淘宝开放平台的接口,根据商品ID获取商品信息
const res = await fetch(`https://api.taobao.com/rest/api3.do?method=taobao.item.get&appkey=xxx&secret=xxx&num_iid=${productId}`)
const data = await res.json()
// 将商品信息添加到足迹列表中
commit('addFootprint', {
id: data.item.id,
name: data.item.title,
img: data.item.pic_url,
price: data.item.price
})
}
}
})
```
3. 在路由文件中添加详情页的路由
```javascript
import Vue from 'vue'
import Router from 'vue-router'
import Footprint from '@/components/Footprint'
import Detail from '@/components/Detail'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Footprint',
component: Footprint
},
{
path: '/detail/:id',
name: 'Detail',
component: Detail
}
]
})
```
4. 在详情页组件 Detail.vue 中显示商品详情信息
```html
<template>
<div>
<h1>{{ item.name }}</h1>
<img :src="item.img" alt="">
<p>{{ item.price }}</p>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState(['footprintList']),
item() {
return this.footprintList.find(item => item.id === this.$route.params.id)
}
}
}
</script>
```
以上是一个简单的 Vue 淘宝足迹功能的代码实现,您可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
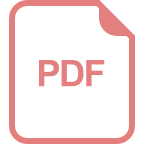
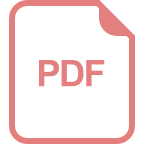
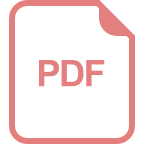
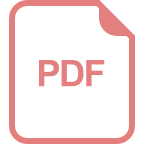
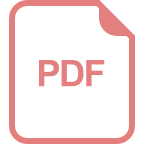
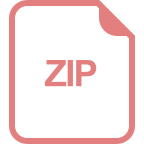
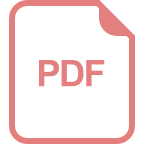
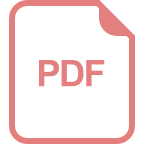
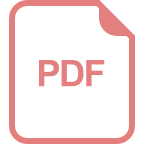
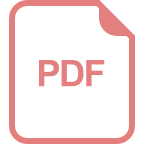
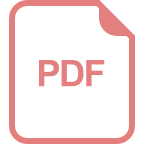
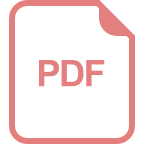
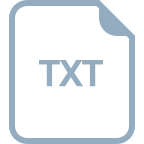