idea 中jsp页面实现验证码登陆
时间: 2024-03-18 18:39:54 浏览: 92
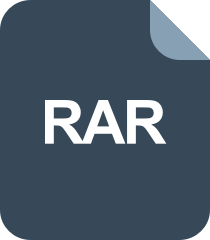
jsp 登陆页面验证码的实现

以下是使用 JSP 页面实现验证码登录的示例代码:
1.创建一个Controller类:
```java
@Controller
public class LoginController {
private static final String SESSION_KEY_CHECKCODE = "checkCode";
@GetMapping("/login")
public String login() {
return "login";
}
@PostMapping("/login")
public String login(String username, String password, String checkCode, HttpSession session, Model model) {
// 验证码校验
String checkCodeSession = (String) session.getAttribute(SESSION_KEY_CHECKCODE);
if (!checkCode.equalsIgnoreCase(checkCodeSession)) {
model.addAttribute("errorMsg", "验证码错误");
return "login";
}
// 用户名和密码校验
if ("admin".equals(username) && "123456".equals(password)) {
// 登录成功
return "redirect:/success";
} else {
// 登录失败
model.addAttribute("errorMsg", "用户名或密码错误");
return "login";
}
}
@GetMapping("/checkCode")
public void checkCode(HttpServletRequest request, HttpServletResponse response, HttpSession session) throws IOException {
// 定义图片宽、高
int width = 100;
int height = 50;
// 创建 BufferedImage 对象,设置图片大小和类型
BufferedImage img = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
// 获取 Graphics2D 对象,用于绘制图形
Graphics2D graphics2D = img.createGraphics();
// 设置背景色
graphics2D.setColor(Color.WHITE);
graphics2D.fillRect(0, 0, width, height);
// 设置字体
graphics2D.setFont(new Font("宋体", Font.BOLD, 20));
// 随机生成 4 位验证码
StringBuilder sb = new StringBuilder();
Random random = new Random();
for (int i = 0; i < 4; i++) {
int num = random.nextInt(10);
sb.append(num);
graphics2D.setColor(new Color(random.nextInt(255), random.nextInt(255), random.nextInt(255)));
graphics2D.drawString(String.valueOf(num), i * 20, 30);
}
// 将验证码存入 session 中
session.setAttribute(SESSION_KEY_CHECKCODE, sb.toString());
// 释放 Graphics2D 对象
graphics2D.dispose();
// 将验证码图片输出到浏览器
ImageIO.write(img, "JPEG", response.getOutputStream());
}
@GetMapping("/success")
public String success() {
return "success";
}
}
```
2.创建一个login.jsp页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>验证码登录</title>
</head>
<body>
<h2>验证码登录</h2>
<form method="post" action="/login">
<label for="username">用户名:</label>
<input type="text" id="username" name="username"/><br/>
<label for="password">密码:</label>
<input type="password" id="password" name="password"/><br/>
<label for="checkCode">验证码:</label>
<input type="text" id="checkCode" name="checkCode"/>
<img src="/checkCode"/><br/>
<input type="submit" value="登录"/>
</form>
<div style="color: red">${errorMsg}</div>
</body>
</html>
```
3.创建一个success.jsp页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>登录成功</title>
</head>
<body>
<h2>登录成功</h2>
</body>
</html>
```
4.运行代码,在浏览器中访问 "http://localhost:8080/login" 即可看到验证码登录页面。
注意:此示例仅包含基本的验证码登录功能,实际应用中需要添加身份验证、安全性等方面的特性。
阅读全文
相关推荐
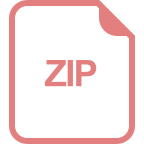
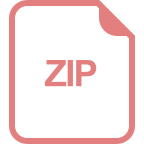
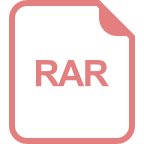
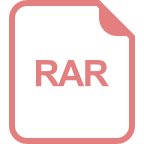
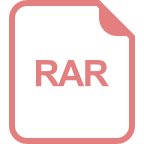
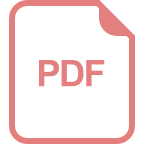
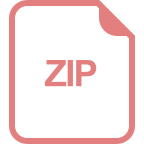
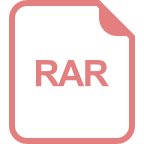
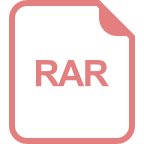
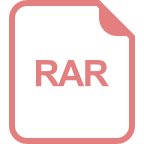
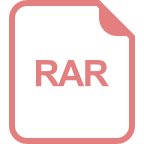
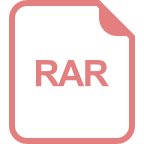
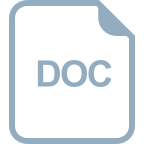
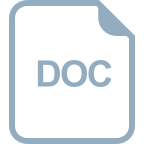
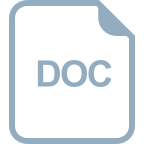
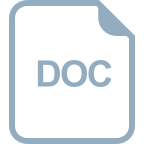