给一非空的单词列表,返回前 k 个出现次数最多的单词。 返回的答案应该按单词出现频率由高到低排序。如果不同的单词有相同出现频率,按字母顺序排序。 输入:第1行为k值,第2行单词列表,单词列表以end结尾,end不属于单词列表中的单词。
时间: 2024-02-03 13:14:16 浏览: 113
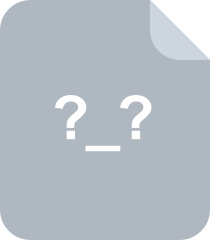
单词的词频统计,按出现的频率递减的次序输出
好的,这是一个很经典的问题,可以使用哈希表和堆来解决。我们首先需要统计每个单词出现的次数,然后按照频率和字母顺序排序。可以先使用哈希表来统计每个单词出现的次数,然后将哈希表中的元素插入一个小根堆中,堆的大小为k,保证堆中始终只有前k个出现次数最多的单词。最后对堆中的元素按照频率和字母顺序进行排序,即可得到最终结果。
下面是一份Python代码实现:
```python
import heapq
from collections import defaultdict
def topKFrequent(words, k):
freq = defaultdict(int)
for word in words:
freq[word] += 1
heap = []
for word, count in freq.items():
heapq.heappush(heap, WordCount(word, count, -ord(word)))
if len(heap) > k:
heapq.heappop(heap)
res = []
while heap:
res.append(heapq.heappop(heap).word)
return res[::-1]
class WordCount:
def __init__(self, word, count, order):
self.word = word
self.count = count
self.order = order
def __lt__(self, other):
if self.count != other.count:
return self.count < other.count
else:
return self.order > other.order
words = []
while True:
word = input()
if word == "end":
break
words.append(word)
k = int(input())
res = topKFrequent(words, k)
print(res)
```
我们定义了一个名为`WordCount`的类来存储单词的出现次数和字母顺序。在比较两个`WordCount`对象时,首先按照出现次数排序,如果出现次数相同,则按照字母顺序进行排序。
在主函数中,我们首先使用`defaultdict`来统计每个单词的出现次数,然后将其插入小根堆中。我们还需要注意,如果堆的大小超过了k,我们需要弹出堆顶元素,以保证堆中只有前k个出现次数最多的单词。
最后,我们将堆中的元素按照出现次数和字母顺序进行排序,并返回最终结果。
希望对你有帮助!
阅读全文
相关推荐
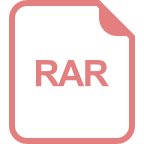






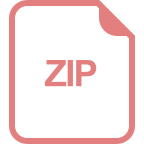
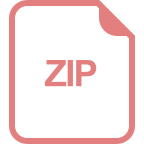
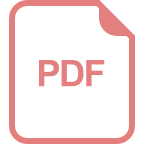
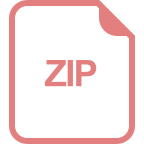
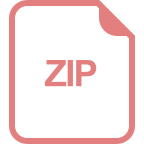
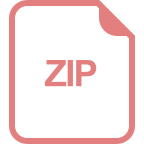
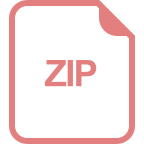
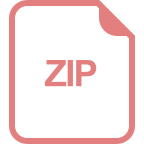
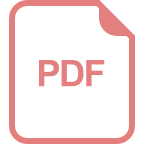
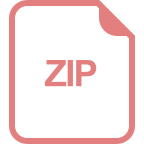