springboot树形结构示例
时间: 2023-06-30 12:21:59 浏览: 118
以下是一个使用Spring Boot实现树形结构的示例:
1. 定义实体类
```
@Entity
@Table(name = "category")
public class Category {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "parent_id")
private Category parent;
@OneToMany(mappedBy = "parent", cascade = CascadeType.ALL, orphanRemoval = true)
private Set<Category> children = new HashSet<>();
// constructors, getters and setters
}
```
2. 定义Repository接口
```
@Repository
public interface CategoryRepository extends JpaRepository<Category, Long> {
List<Category> findByParentIsNull();
}
```
3. 定义Service接口和实现类
```
@Service
public interface CategoryService {
List<Category> getAllCategories();
}
@Service
public class CategoryServiceImpl implements CategoryService {
@Autowired
private CategoryRepository categoryRepository;
@Override
public List<Category> getAllCategories() {
List<Category> categories = categoryRepository.findByParentIsNull();
for (Category category : categories) {
fetchChildren(category);
}
return categories;
}
private void fetchChildren(Category category) {
Set<Category> children = category.getChildren();
if (!children.isEmpty()) {
for (Category child : children) {
fetchChildren(child);
}
}
}
}
```
4. 定义Controller类
```
@RestController
@RequestMapping("/categories")
public class CategoryController {
@Autowired
private CategoryService categoryService;
@GetMapping
public List<Category> getAllCategories() {
return categoryService.getAllCategories();
}
}
```
这个示例中,我们使用了一个自关联的实体类Category来表示树形结构,通过Category的parent和children属性来维护父子关系。在CategoryRepository中,我们定义了一个findByParentIsNull方法来获取所有的根节点。在CategoryServiceImpl中,我们通过递归的方式来获取所有的子节点,并将它们作为树形结构返回给Controller。最后,在CategoryController中,我们将树形结构返回给客户端。
阅读全文
相关推荐
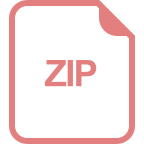

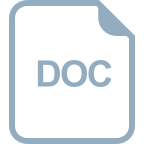





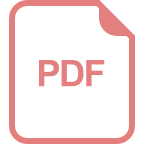
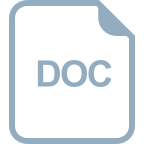



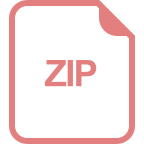
