springboot树形结构条件查询
时间: 2023-07-20 15:22:34 浏览: 58
树形结构条件查询是指对于一棵树结构,根据指定的条件查询出符合条件的节点。在Spring Boot中实现树形结构条件查询可以使用递归的方式进行查询,以下是一个示例:
假设我们有一个部门表,其中有id、name、parentId三个字段,parentId表示当前部门的父级部门ID,如果为0则表示当前部门为顶级部门。我们需要实现根据指定的部门ID查询出该部门及其所有子部门信息的功能。
首先,我们需要定义一个Department实体类,包含id、name、parentId三个字段,并通过JPA注解将其映射到数据库表中。
```java
@Entity
@Table(name = "department")
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(name = "parent_id")
private Long parentId;
// getter and setter
}
```
接下来,我们定义一个DepartmentRepository接口,继承JpaRepository接口,并定义一个findByParentId方法,用于递归查询当前部门及其所有子部门信息。
```java
@Repository
public interface DepartmentRepository extends JpaRepository<Department, Long> {
List<Department> findByParentId(Long parentId);
}
```
在上述方法中,我们使用了Spring Data JPA提供的findByParentId方法,并通过递归方式查询出当前部门及其所有子部门信息。
最后,我们定义一个DepartmentService类,注入DepartmentRepository接口,并定义一个findDepartmentTree方法,用于查询指定部门及其所有子部门信息。
```java
@Service
public class DepartmentService {
@Autowired
private DepartmentRepository departmentRepository;
public List<Department> findDepartmentTree(Long departmentId) {
List<Department> departments = departmentRepository.findByParentId(departmentId);
for (Department department : departments) {
department.setChildren(findDepartmentTree(department.getId()));
}
return departments;
}
}
```
在上述方法中,我们首先通过DepartmentRepository中的findByParentId方法查询出当前部门的所有子部门信息,然后通过递归方式对每个子部门再次调用findDepartmentTree方法,查询出其所有子部门信息,并设置到当前部门的children属性中,最终返回所有部门信息的列表。
通过上述示例,我们实现了树形结构条件查询的功能,可以根据指定的部门ID查询出该部门及其所有子部门信息。
相关推荐
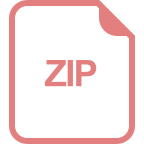














