我在Qt中编写了两个cpp文件,分别命名为dialog.cpp与form.cpp,dialog.cpp的功能是用来接收串口数据的,现在需要你编写一份代码,使dialog.cpp内的串口所接收到的数据,可以在form.cpp中的LineEdit内被读取出来
时间: 2023-09-26 08:06:50 浏览: 163
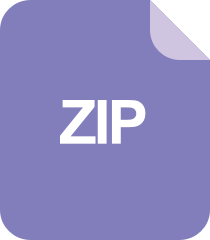
QT实现十六机制接收串口程序,自己写的代码,打开编译就能用!!!
### 回答1:
要实现这个功能,你需要在 `dialog.cpp` 中将接收到的串口数据发送到 `form.cpp` 中。具体实现步骤如下:
1. 在 `dialog.h` 中添加一个信号 `serialDataReceived(QString data)`,用于在串口数据接收到时发出信号。
```cpp
signals:
void serialDataReceived(QString data);
```
2. 在 `dialog.cpp` 中,当串口数据接收到时,通过 `emit` 发送信号 `serialDataReceived` 并传递数据。
```cpp
void Dialog::serialReceived()
{
QByteArray data = serialPort->readAll();
QString str(data);
emit serialDataReceived(str);
}
```
3. 在 `form.cpp` 中,连接 `dialog` 对象的 `serialDataReceived` 信号到一个槽函数中,该槽函数用于在 `LineEdit` 中显示接收到的数据。
```cpp
connect(dialog, &Dialog::serialDataReceived, this, &Form::showReceivedData);
```
4. 在 `form.h` 中添加一个槽函数 `showReceivedData(QString data)`,用于将接收到的数据显示在 `LineEdit` 中。
```cpp
public slots:
void showReceivedData(QString data);
```
5. 在 `form.cpp` 中实现 `showReceivedData` 槽函数,将接收到的数据显示在 `LineEdit` 中。
```cpp
void Form::showReceivedData(QString data)
{
ui->lineEdit->setText(data);
}
```
这样,当串口数据接收到时,`dialog.cpp` 会发出 `serialDataReceived` 信号,`form.cpp` 中的槽函数 `showReceivedData` 就会被调用,将数据显示在 `LineEdit` 中。
### 回答2:
在Qt中,可以通过信号与槽机制来实现dialog.cpp与form.cpp之间的数据传递。以下是一份代码示例,实现了你的需求:
在dialog.cpp中,我们定义一个信号sendData,用于将接收到的串口数据发送给form.cpp:
```cpp
// dialog.cpp
#include "dialog.h"
#include "ui_dialog.h"
Dialog::Dialog(QWidget *parent) :
QDialog(parent),
ui(new Ui::Dialog)
{
ui->setupUi(this);
// 创建一个 QSerialPort 对象,用于串口通信
serialPort = new QSerialPort(this);
// 配置串口参数
// ...
// 连接串口的readyRead()信号到我们自定义的槽函数readData()
connect(serialPort, &QSerialPort::readyRead, this, &Dialog::readData);
}
Dialog::~Dialog()
{
delete ui;
}
void Dialog::readData()
{
// 读取串口数据
QByteArray data = serialPort->readAll();
// 发送串口数据给form.cpp
emit sendData(data);
}
```
在form.cpp中,我们通过连接信号与槽,将接收到的串口数据显示在LineEdit控件中:
```cpp
// form.cpp
#include "form.h"
#include "ui_form.h"
Form::Form(QWidget *parent) :
QWidget(parent),
ui(new Ui::Form)
{
ui->setupUi(this);
// 连接dialog.cpp的sendData信号到我们自定义的槽函数updateData()
connect(&dialog, &Dialog::sendData, this, &Form::updateData);
}
Form::~Form()
{
delete ui;
}
void Form::updateData(const QByteArray &data)
{
// 将接收到的串口数据显示在LineEdit中
ui->lineEdit->setText(data);
}
```
在主函数中,我们创建Dialog和Form对象,并显示它们:
```cpp
#include "dialog.h"
#include "form.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Dialog dialog;
Form form;
dialog.show();
form.show();
return a.exec();
}
```
通过以上代码,dialog.cpp内接收到的串口数据会被发送到form.cpp中的LineEdit内,实现了数据的传递。请注意,以上代码仅是示例,实际情况下可能需要根据具体需求做适当的修改和调整。
### 回答3:
你可以使用信号和槽机制来实现dialog.cpp内的串口数据在form.cpp中的LineEdit中被读取出来。
首先,在dialog.cpp中定义一个信号,用来传递串口接收到的数据。你可以在dialog.cpp的头文件中添加如下代码:
```
signals:
void dataReceived(const QString& data);
```
然后,在dialog.cpp中接收串口数据的地方,使用emit语句来发射信号,将接收到的数据传递出去。假设你在dialog.cpp中有一个叫做dataReceived()的函数,用来接收串口数据,你可以在该函数中添加如下代码:
```
void Dialog::dataReceived(const QByteArray& data)
{
// 将接收到的数据转换为QString类型
QString receivedData = QString::fromUtf8(data);
// 发射信号,将数据传递到form.cpp中
emit dataReceived(receivedData);
}
```
接着,在form.cpp中,连接dialog.cpp的信号和LineEdit的槽函数,以便将接收到的数据显示在LineEdit中。你可以在form.cpp的构造函数中添加如下代码:
```
Form::Form(QWidget *parent)
: QWidget(parent)
{
// 创建一个LineEdit对象
QLineEdit *lineEdit = new QLineEdit(this);
// 连接dialog.cpp的dataReceived()信号和LineEdit的setText()槽函数
connect(dialog, &Dialog::dataReceived, lineEdit, &QLineEdit::setText);
}
```
这样,每当dialog.cpp接收到数据时,它会发射dataReceived()信号,传递数据给form.cpp中的LineEdit的setText()槽函数,用来设置LineEdit的文本内容为接收到的数据。
需要注意的是,你需要在form.cpp中访问到dialog.cpp的实例,以便进行信号和槽的连接。你可以在form.cpp中添加一个成员变量来保存dialog.cpp的实例,并在构造函数或者其他地方将其赋值。
阅读全文
相关推荐
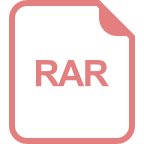
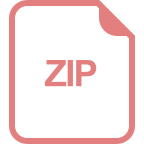
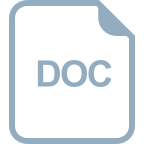
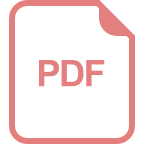
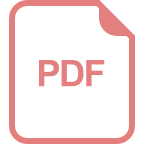
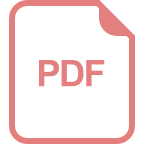
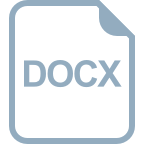
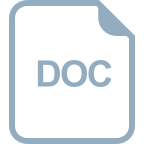
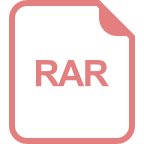
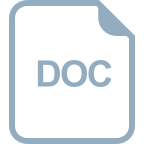
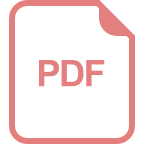
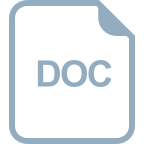
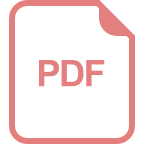
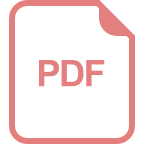
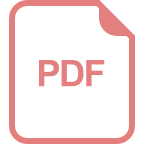
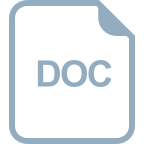
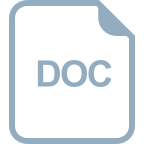
