帮我写一个HOG特征和极大值抑制的行人检测python代码
时间: 2024-05-12 14:18:12 浏览: 111
好的,以下是Python代码的实现:
```python
import cv2
# HOG参数
winSize = (64, 128)
blockSize = (16, 16)
blockStride = (8, 8)
cellSize = (8, 8)
nbins = 9
# 加载行人检测模型
hog = cv2.HOGDescriptor(winSize, blockSize, blockStride, cellSize, nbins)
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# 极大值抑制参数
threshold = 0.3
max_overlap = 0.5
# 行人检测函数
def detect_person(image):
# 运行HOG检测器
rects, weights = hog.detectMultiScale(image)
# 极大值抑制
if len(rects) > 1:
weights = weights[:, 0]
rects = rects[np.argsort(-weights)]
keep = []
while len(rects) > 0:
x1, y1, w1, h1 = rects[0]
keep.append(x1, y1, x1 + w1, y1 + h1)
overlaps = compute_overlap(rects[1:], [x1, y1, x1 + w1, y1 + h1])
inds = np.where(overlaps <= max_overlap)[0]
rects = rects[inds + 1]
return np.array(keep)
else:
return rects
# 计算两个矩形框之间的IOU(交并比)
def compute_overlap(rects, bbox):
x1 = np.maximum(rects[:, 0], bbox[0])
y1 = np.maximum(rects[:, 1], bbox[1])
x2 = np.minimum(rects[:, 2], bbox[2])
y2 = np.minimum(rects[:, 3], bbox[3])
inter_area = np.maximum(x2 - x1, 0) * np.maximum(y2 - y1, 0)
bbox_area = (bbox[2] - bbox[0]) * (bbox[3] - bbox[1])
overlap = inter_area / bbox_area
return overlap
```
使用方法:
```python
# 加载图像
image = cv2.imread('person.jpg')
# 行人检测
rects = detect_person(image)
# 绘制检测结果
for (x, y, w, h) in rects:
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示结果图像
cv2.imshow('detection result', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,这只是一个简单的示例,可能不是最优的行人检测算法。请根据实际需求进行修改和改进。
阅读全文
相关推荐
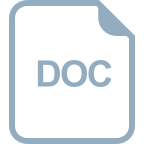
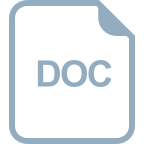
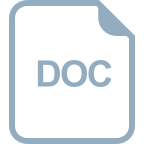

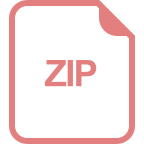
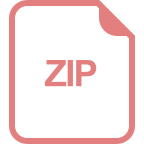
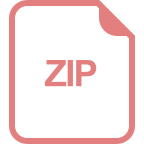
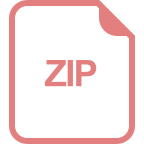
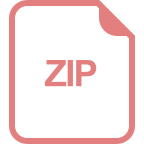
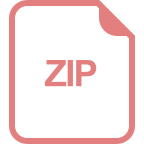
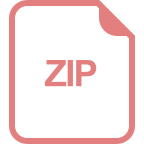
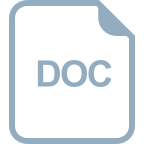
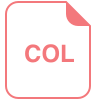
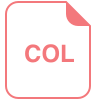
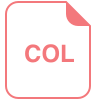
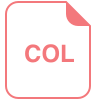
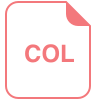
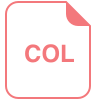
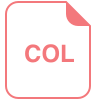