如何利用定时器 3 的通道 2 实现呼吸灯效果?
时间: 2024-11-29 18:37:00 浏览: 14
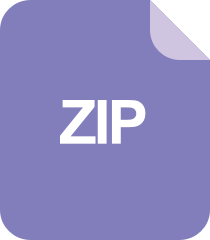
TIM—全彩呼吸灯
要在Arduino或类似平台通过定时器3的通道2实现呼吸灯效果,首先你需要了解一些基本概念:
1. **定时器3**:通常用于处理精确的时间间隔任务,比如LED闪烁。Arduino Uno或类似板子上,Timer3是一个8位定时器。
2. **通道2**:Timer3有两个工作通道,可以设置独立的计数器和中断服务程序(ISRs),用于控制不同的动作。
下面是简单的步骤:
- **初始化定时器3**:需要设置定时器的工作模式(如方波输出、PWM等)、分频器值、以及启动定时器。
```c++
#include <TimerOne.h>
TimerOne.initialize(OCR1A, OCR1B); // OCR1A and OCR1B for channels 0 and 1 (channel 2 is not directly available)
TimerOne.setMode(TIMER1_MODE_PWM, PWM_CENTRE_ALIGNED);
```
- **创建呼吸频率变量**:确定LED闪烁的速度,可以作为周期或频率。
```c++
int breathFrequency = 50; // Breath cycle frequency in milliseconds
```
- **编写呼吸函数**:该函数会改变LED亮度(通常是通过调整PWM duty cycle)来模拟呼吸效果。
```c++
void breathe(int brightness) {
analogWrite(LED_PIN, brightness);
}
```
- **设置定时器中断**:每当定时器溢出时,调用breath函数,并更新亮度值(例如每次降低或增加一点)。
```c++
attachInterrupt(digitalPinToInterrupt(TIMER3_PIN), changeBrightness, RISING); // TIMER3_PIN is the interrupt pin for Timer 3 channel 2
void changeBrightness() {
static bool decrease = true;
if (decrease) {
// Decrease brightness
breathe(brightness - 1);
if (brightness <= 0) { // If brightness reaches zero, switch direction
decrease = false;
}
} else {
// Increase brightness
breathe(brightness + 1);
if (brightness >= MAX_BRIGHTNESS) { // If brightness reaches max, switch back to decrease
decrease = true;
}
}
}
```
- **启动定时器和中断**:
```c++
TimerThree.start();
changeBrightness(); // Initial call to start the breathing effect
```
记住,这只是一个基础示例,实际应用中可能还需要考虑延时、异常处理等问题。此外,由于Timer3的限制,你可能需要外部中断或者其他方法间接使用Channel2。如果你正在使用的硬件支持的话。
阅读全文
相关推荐
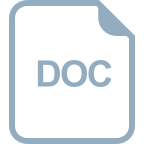
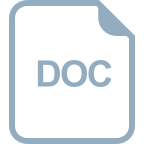

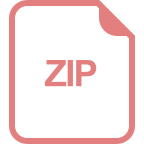
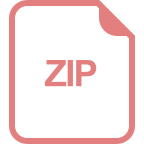
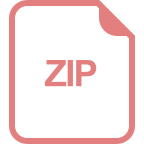
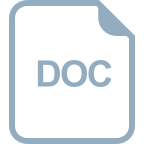
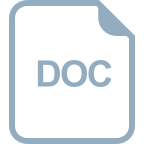









