from sklearn.metrics import auc,roc_curve def evaluation_class(model, x_test, y_test): prediction = model.predict_proba(x_test) preds = model.predict_proba(x_test)[:, 1] fpr,tpr,threshold = roc_curve(y_test,preds) roc_auc = auc(fpr,tpr) plt.title('ROC Curve') plt.plot(fpr,tpr,'g',label = 'AUC = %0.3f' % roc_auc) plt.legend(loc = 'lower right') plt.plot([0,1],[0,1],'r--') plt.xlim([0,1]) plt.ylim([0,1]) plt.ylabel('True Positive Rate') plt.xlabel('False Positive Rate') plt.show() print('ROC AUC score:', round(roc_auc, 4)) from sklearn.ensemble import RandomForestClassifier from sklearn.linear_model import LogisticRegression from sklearn.preprocessing import StandardScaler from sklearn import svm x_train = StandardScaler().fit_transform(x_train) x_test = StandardScaler().fit_transform(x_test) lr = LogisticRegression() lr.fit(x_train,y_train) evaluation_class(lr,x_test,y_test) rf=RandomForestClassifier(max_depth=2,random_state=0) rf.fit(x_train,y_train) evaluation_class(rf,x_test,y_test) sm = svm.SVC(gamma='scale',C=1.0,decision_function_shape='ovr',kernel='rbf',probability=True) sm.fit(x_train,y_train) evaluation_class(sm,x_test,y_test)
时间: 2024-04-05 19:33:57 浏览: 15
这是一个Python代码片段,用于评估分类模型的性能,并使用三种不同的分类算法(逻辑回归、随机森林和支持向量机)进行训练和测试。具体来说,代码定义了一个evaluation_class函数,用于计算和绘制ROC曲线,并输出ROC AUC得分。然后,代码使用sklearn库中的StandardScaler函数,对训练集和测试集进行标准化处理。接下来,代码使用sklearn库中的LogisticRegression、RandomForestClassifier和svm.SVC函数,分别训练三种不同的分类算法,并使用evaluation_class函数对每个模型进行评估。
在evaluation_class函数中,代码使用模型的predict_proba函数,对测试集进行预测,并计算ROC曲线的FPR和TPR。然后,代码使用sklearn库中的auc函数,计算ROC曲线下的面积,并将ROC曲线和AUC值绘制在图表上。最后,代码输出ROC AUC得分。
总体来说,这段代码用于比较不同的分类算法在给定数据集上的性能表现,通过计算ROC AUC曲线和得分,评估模型的分类准确度和稳定性。
相关问题
fpr, tpr, thresholds = metrics.roc_curve(y_test, prediction)
这行代码使用了scikit-learn中的metrics模块,其中的roc_curve函数用于计算ROC曲线的参数。具体来说,它接受两个参数:y_test是测试集的真实标签,prediction是模型对测试集样本的预测结果(通常是概率或者分数)。函数的返回值是三个NumPy数组,分别代表了不同阈值下的假正率(false positive rate,FPR)、真正率(true positive rate,TPR)和对应的阈值(thresholds)。通过这些参数,我们可以绘制ROC曲线并计算AUC(Area Under Curve,曲线下面积),以评估模型的分类性能。
import networkx as nxfrom sklearn.model_selection import train_test_splitfrom networkx.algorithms.link_prediction import katz_similarityfrom sklearn.metrics import roc_auc_score, average_precision_score# 加载数据集edges = []with open("email-Eu-core.txt") as f: for line in f: if line.startswith("#"): continue edge = line.strip().split() edges.append((int(edge[0]), int(edge[1])))# 划分训练集和测试集train_edges, test_edges = train_test_split(edges, test_size=0.1, random_state=42)# 构建无向图graph = nx.Graph()graph.add_edges_from(train_edges)# 计算相似度katz_scores = katz_similarity(graph, max_l=200, beta=0.01)# 计算AUC值y_true = [1 if edge in test_edges else 0 for edge in graph.edges()]y_scores = [katz_scores[edge] for edge in graph.edges()]auc = roc_auc_score(y_true, y_scores)# 计算Precisionk = 100top_k_edges = sorted(graph.edges(), key=lambda x: katz_scores[x], reverse=True)[:k]y_true = [1 if edge in test_edges else 0 for edge in top_k_edges]y_scores = [katz_scores[edge] for edge in top_k_edges]precision = average_precision_score(y_true, y_scores)print("Katz Algorithm")print("AUC: {:.4f}".format(auc))print("Precision@{}: {:.4f}".format(k, precision))有什么问题
这段代码中有一个语法错误,即第一行的 import 语句中没有正确地使用换行符。应该在 `import networkx as nx` 后添加一个换行符。
另外,代码中的 katz_similarity() 函数调用中使用了一个 max_l 参数,这个参数是不必要的,可以直接去掉,因为该函数不支持传递该参数。
最后,代码未定义变量 k,所以在计算 Precision 时会出错。您应该在代码开头定义一个 k 变量,或者将 k 的值硬编码到代码中。
下面是修改后的代码:
```python
import networkx as nx
from sklearn.model_selection import train_test_split
from networkx.algorithms.link_prediction import katz_similarity
from sklearn.metrics import roc_auc_score, average_precision_score
# 加载数据集
edges = []
with open("email-Eu-core.txt") as f:
for line in f:
if line.startswith("#"):
continue
edge = line.strip().split()
edges.append((int(edge[0]), int(edge[1])))
# 划分训练集和测试集
train_edges, test_edges = train_test_split(edges, test_size=0.1, random_state=42)
# 构建无向图
graph = nx.Graph()
graph.add_edges_from(train_edges)
# 计算相似度
katz_scores = katz_similarity(graph, beta=0.01)
# 计算AUC值
y_true = [1 if edge in test_edges else 0 for edge in graph.edges()]
y_scores = [katz_scores[edge] for edge in graph.edges()]
auc = roc_auc_score(y_true, y_scores)
# 计算Precision
k = 100
top_k_edges = sorted(graph.edges(), key=lambda x: katz_scores[x], reverse=True)[:k]
y_true = [1 if edge in test_edges else 0 for edge in top_k_edges]
y_scores = [katz_scores[edge] for edge in top_k_edges]
precision = average_precision_score(y_true, y_scores)
print("Katz Algorithm")
print("AUC: {:.4f}".format(auc))
print("Precision@{}: {:.4f}".format(k, precision))
```
请注意,这段代码依赖于 `email-Eu-core.txt` 数据集文件,您需要确保该文件存在并且与代码在同一目录下。
相关推荐
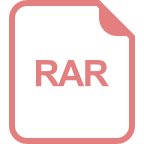











