int size(LNode *stack) { //获得链栈中结点个数 LNode *topNode = stack->next; int len = while (topNode != NULL) { LNode *temp = topNode->next; //先保存后继指针 free(topNode); topNode = temp; } stack->next = NULL; } void destroy(LNode **stack) { // 释放头结点空间 free(*stack); } int main(){ //由同学编写代码 如何完善
时间: 2023-06-14 13:06:16 浏览: 96
完善的代码应该是这样的:
#include <stdio.h>
#include <stdlib.h>
typedef struct LNode {
int data;
struct LNode *next;
} LNode;
void push(LNode **stack, int data) {
LNode *newNode = (LNode *)malloc(sizeof(LNode));
newNode->data = data;
newNode->next = *stack;
*stack = newNode;
}
int pop(LNode **stack) {
if (*stack == NULL) {
printf("栈已空,无法弹出元素!\n");
return 0;
}
int data = (*stack)->data;
LNode *temp = *stack;
*stack = (*stack)->next;
free(temp);
return data;
}
int size(LNode *stack) {
LNode *topNode = stack->next;
int len = 0;
while (topNode != NULL) {
len++;
topNode = topNode->next;
}
return len;
}
void destroy(LNode **stack) {
while (*stack != NULL) {
pop(stack);
}
free(*stack); // 释放头结点空间
*stack = NULL;
}
int main() {
LNode *stack = (LNode *)malloc(sizeof(LNode));
stack->next = NULL;
push(&stack, 1);
push(&stack, 2);
push(&stack, 3);
printf("栈大小为:%d\n", size(stack));
printf("弹出元素:%d\n", pop(&stack));
printf("弹出元素:%d\n", pop(&stack));
printf("弹出元素:%d\n", pop(&stack));
printf("栈大小为:%d\n", size(stack));
destroy(&stack);
return 0;
}
这段代码实现了链栈的基本操作,包括入栈(push)、出栈(pop)、获取栈大小(size)和销毁栈(destroy)。在主函数中,我们先创建了一个空的链栈,然后依次将元素1、2、3入栈,再依次弹出元素,并获取栈大小,最后销毁栈。
阅读全文
相关推荐
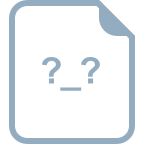
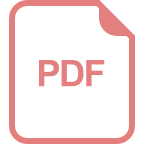
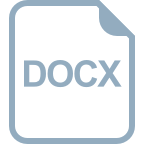

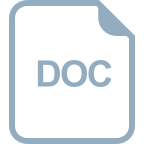
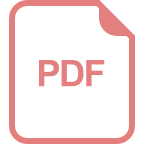
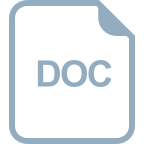
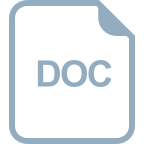
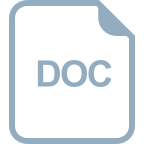
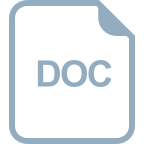





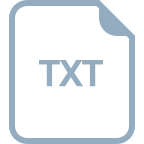
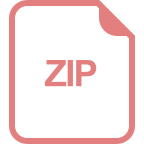
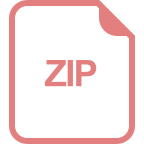
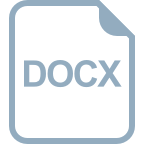
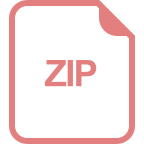