、需求:用Set集合存储自定义对象并遍历Student(name,age) 分析: A:创建学生类 B:创建集合对象 C:创建学生对象 D:把学生对象添加到集合对象中 E:遍历集合(使用迭代器和增强for两种遍历方式)
时间: 2024-02-07 21:04:11 浏览: 34
好的,让我来回答你的问题。
首先,您需要创建一个`Student`类,该类应该至少包含`name`和`age`两个属性,并且需要提供一个构造函数用于创建对象。
接下来,您需要创建一个`Set`集合对象来存储`Student`对象。`Set`集合是一个不允许重复元素的集合,所以您需要在添加`Student`对象之前检查该对象是否已经存在于集合中。
以下是实现该需求的示例代码:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
// 创建Set集合对象
Set<Student> set = new HashSet<>();
// 创建Student对象
Student s1 = new Student("Tom", 18);
Student s2 = new Student("Jerry", 20);
Student s3 = new Student("Alice", 19);
// 把Student对象添加到Set集合中
set.add(s1);
set.add(s2);
set.add(s3);
// 遍历Set集合(使用迭代器)
Iterator<Student> it = set.iterator();
while (it.hasNext()) {
Student s = it.next();
System.out.println(s.getName() + " " + s.getAge());
}
// 遍历Set集合(使用增强for)
for (Student s : set) {
System.out.println(s.getName() + " " + s.getAge());
}
}
}
class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (obj instanceof Student) {
Student s = (Student) obj;
if (this.name.equals(s.getName()) && this.age == s.getAge()) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
```
在上面的代码中,我们重写了`Student`类的`equals()`和`hashCode()`方法,这是因为`Set`集合在判断元素是否重复时,会调用元素的`equals()`和`hashCode()`方法。我们只需要判断`Student`对象的`name`和`age`属性是否相同即可。
相关推荐
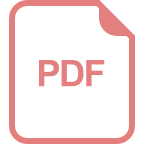
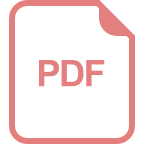











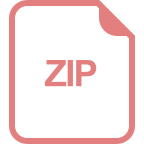
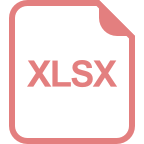
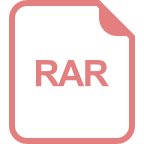
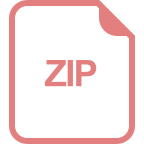